一元多项式c语言代码
时间: 2024-09-24 08:05:46 浏览: 30
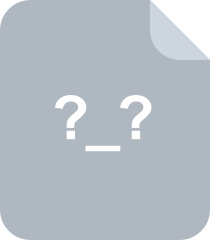
一元稀疏多项式运算代码

在C语言中,一元多项式通常表示为形式如`a_n*x^n + a_{n-1}*x^(n-1) + ... + a_0`的形式,其中`a_i`是系数,`x`是变量,`n`是非负整数。下面是一个简单的C语言代码片段,用于表示和操作一元多项式的实例:
```c
#include <stdio.h>
// 定义结构体表示多项式的项
typedef struct {
double coefficient; // 系数
int exponent; // 指数
} PolynomialTerm;
// 结构体数组来存储多项式
typedef PolynomialTerm Term[10]; // 假设最多有10项
// 表示多项式函数
typedef void (*Polynomial)(Term[], int terms_count, double x);
// 乘法运算(假设两个多项式相乘)
void multiply(Term result[], int& result_terms_count, const Term poly1[], int poly1_count, const Term poly2[], int poly2_count) {
for (int i = 0; i <= poly1_count + poly2_count - 1; ++i) {
result[i].coefficient = 0;
result[i].exponent = 0;
for (int j = 0; j < poly1_count && i - j >= 0; ++j) {
result[i].coefficient += poly1[j].coefficient * poly2[i - j].coefficient;
result[i].exponent += poly1[j].exponent + poly2[i - j].exponent;
}
if (result[i].exponent > 0)
result_terms_count = i + 1;
}
}
// 主函数演示多项式计算
int main() {
Term poly1 = { {1.0, 2}, {0.5, 1} }; // a + 0.5x
Term poly2 = { {2.0, 3}, {1.0, 1} }; // 2x^3 + x
int poly1_terms_count = 2;
int poly2_terms_count = 2;
Term result(10); // 初始化结果数组
int result_terms_count = 0;
multiply(result, result_terms_count, poly1, poly1_terms_count, poly2, poly2_terms_count);
printf("Result polynomial: ");
for (int i = 0; i < result_terms_count; ++i) {
if (result[i].exponent == 0)
printf("%.1f", result[i].coefficient);
else
printf("%.1fx^%d ", result[i].coefficient, result[i].exponent);
}
return 0;
}
```
这个例子展示了如何创建一元多项式,并通过内积的方式模拟了简单的一次多项式乘法。请注意,这只是一个基础版本,实际应用中可能需要处理更复杂的情况,例如合并相同指数的项。
阅读全文
相关推荐
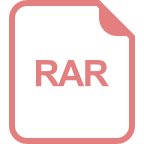
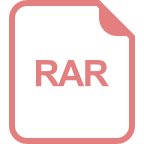
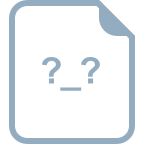
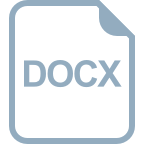
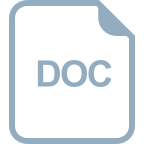
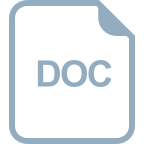
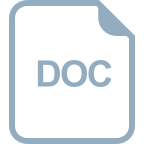









