C语言一元多项式相加的完整代码
时间: 2024-10-25 08:00:32 浏览: 86
在C语言中,我们可以使用数组结构来表示一元多项式,并通过迭代的方式实现两个多项式的相加。以下是一个简单的示例代码:
```c
#include <stdio.h>
// 定义多项式结构体
typedef struct {
int degree; // 次数
double coefficients[100]; // 系数数组,最大次数为99
} Polynomial;
// 初始化多项式
void init_poly(Polynomial* poly, int n) {
poly->degree = n - 1;
for (int i = 0; i <= n; i++) {
poly->coefficients[i] = 0;
}
}
// 相加两个多项式
void add_polynomials(Polynomial* poly1, Polynomial* poly2, Polynomial* sum) {
int max_degree = poly1->degree > poly2->degree ? poly1->degree : poly2->degree;
for (int i = 0; i <= max_degree; i++) {
sum->coefficients[i] = poly1->coefficients[i] + poly2->coefficients[i];
}
sum->degree = max_degree + 1; // 因为加了新项,所以度数增加1
}
// 打印多项式
void print_polynomial(Polynomial poly) {
printf("Degree: %d\n", poly.degree);
for (int i = poly.degree; i >= 0; i--) {
printf("x^%d: %.2f\n", i, poly.coefficients[i]);
}
}
int main() {
Polynomial poly1, poly2, sum;
int n = 5; // 设定最高次项为5
// 初始化多项式
init_poly(&poly1, n);
init_poly(&poly2, n);
// 设置多项式的系数
poly1.coefficients[0] = 2.0;
poly1.coefficients[1] = 4.0;
poly1.coefficients[2] = 6.0;
poly1.coefficients[3] = 8.0;
poly1.coefficients[4] = 10.0;
poly2.coefficients[0] = 1.0;
poly2.coefficients[1] = 3.0;
poly2.coefficients[2] = 5.0;
poly2.coefficients[3] = 7.0;
// 相加多项式
add_polynomials(&poly1, &poly2, &sum);
// 打印结果
print_polynomial(sum);
return 0;
}
```
这个程序首先初始化两个多项式并设置它们的系数,然后通过 `add_polynomials` 函数将它们相加,并保存到 `sum` 中。最后打印出相加后的多项式。
阅读全文
相关推荐
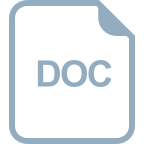
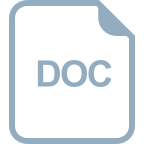
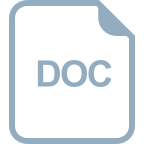
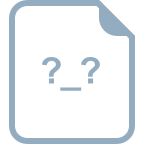







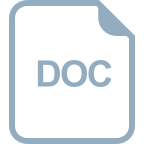
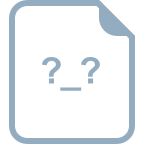
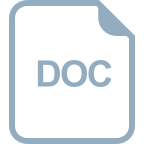
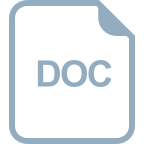


