用C语言写一个将3个线程分配到3个CPU绑核并加锁的代码
时间: 2024-04-15 09:27:16 浏览: 108
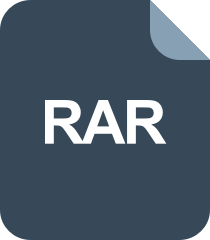
多核线程绑定
在C语言中,线程与CPU核心的绑定是通过设置线程的CPU亲和性来实现的。以下是一个使用C语言编写的将3个线程分配到3个CPU核心并加锁的示例代码:
```c
#define _GNU_SOURCE
#include <stdio.h>
#include <pthread.h>
#include <sched.h>
// 全局变量
int counter = 0;
pthread_mutex_t lock;
// 线程函数
void *thread_function(void *arg) {
int thread_id = *(int*)arg;
// 绑定线程到特定的CPU核心
cpu_set_t cpuset;
CPU_ZERO(&cpuset);
CPU_SET(thread_id, &cpuset);
pthread_setaffinity_np(pthread_self(), sizeof(cpu_set_t), &cpuset);
// 加锁
pthread_mutex_lock(&lock);
printf("Thread %d: Counter before increment: %d\n", thread_id, counter);
counter++;
printf("Thread %d: Counter after increment: %d\n", thread_id, counter);
// 解锁
pthread_mutex_unlock(&lock);
pthread_exit(NULL);
}
int main() {
pthread_t threads[3];
int thread_ids[3] = {0, 1, 2};
// 初始化互斥锁
pthread_mutex_init(&lock, NULL);
// 创建线程
for (int i = 0; i < 3; i++) {
pthread_create(&threads[i], NULL, thread_function, &thread_ids[i]);
}
// 等待线程完成
for (int i = 0; i < 3; i++) {
pthread_join(threads[i], NULL);
}
// 销毁互斥锁
pthread_mutex_destroy(&lock);
return 0;
}
```
在这个示例代码中,我们使用了`pthread_setaffinity_np`函数将每个线程绑定到特定的CPU核心。在`thread_function`中,我们首先设置线程的CPU亲和性,然后进行加锁、操作和解锁操作。
请注意,`#define _GNU_SOURCE`用于启用`CPU_ZERO`和`CPU_SET`宏的定义。另外,这段代码假设有至少3个可用的CPU核心,并且线程的ID与CPU核心的编号是一一对应的。
编译和运行此代码时,请确保使用适当的编译选项,如`-pthread`来链接pthread库,并且具备管理员权限以设置线程的CPU亲和性。
阅读全文
相关推荐
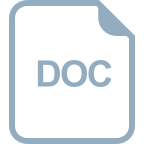

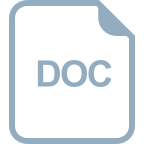
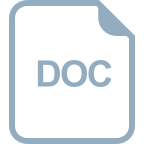
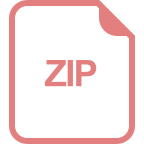
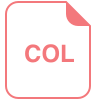
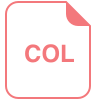
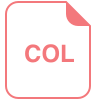
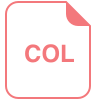
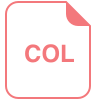
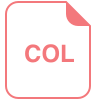
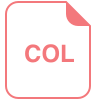
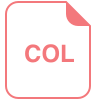
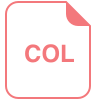
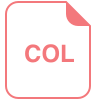
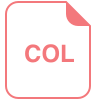
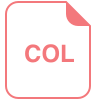