写一个用Unity-Arduino Bluetooth Plugin蓝牙插件开发的代码
时间: 2024-10-19 13:15:37 浏览: 21
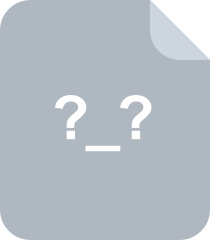
Unity蓝牙通信Arduino Bluetooth Plugin 5.2.1
在Unity中使用Unity-Arduino Bluetooth Plugin蓝牙插件进行开发,首先你需要安装该插件并连接Arduino设备。以下是一个简单的示例,展示如何在Unity脚本中发送数据到Arduino并接收响应:
```csharp
using UnityEngine;
using System.Collections.Generic;
using UnityEngine.UI;
using ArduinoBT;
public class BluetoothManager : MonoBehaviour
{
public Text displayMessage; // UI元素显示接收到的数据
private BluetoothSerial serialPort; // Arduino连接
void Start()
{
// 初始化蓝牙串口通信
serialPort = new BluetoothSerial("COM3"); // 根据你的硬件连接设置对应的端口号
serialPort.Open();
// 启动连接事件监听
serialPort.OnDataReceived += OnDataReceivedFromArduino;
}
void OnDataReceivedFromArduino(byte[] data)
{
string receivedMessage = System.Text.Encoding.ASCII.GetString(data);
displayMessage.text = "Received: " + receivedMessage;
}
// 发送按钮触发的动作
public void SendCommand(string command)
{
byte[] bytes = System.Text.Encoding.ASCII.GetBytes(command);
serialPort.Write(bytes);
}
void Update()
{
if (Input.GetKeyDown(KeyCode.Space)) // 按空格键发送命令
{
SendCommand("Hello from Unity!"); // 发送一个测试字符串
}
}
void OnDestroy()
{
serialPort.Close(); // 游戏关闭时,关闭蓝牙连接
}
}
```
在这个例子中,当你按下空格键,会向Arduino发送一条消息"Hello from Unity!"。然后,Arduino接收到消息后,会在`OnDataReceivedFromArduino`方法中处理并将结果显示在UI文本上。
阅读全文
相关推荐
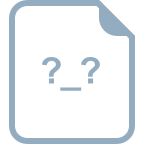

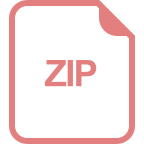

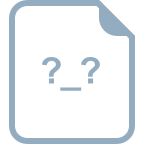
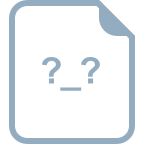


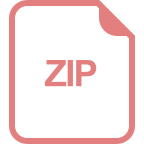
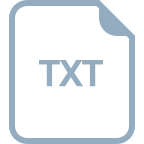

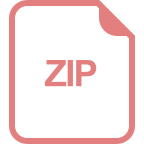
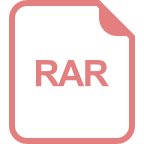