用C++写一个RSA加密算法的程序
时间: 2024-11-07 17:19:29 浏览: 6
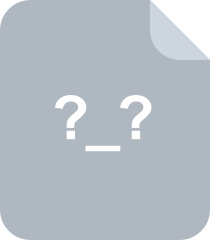
RSA加密算法c++实现
RSA是一种非对称加密算法,其安全性基于大数分解难题。以下是用C++编写一个基本的RSA加密和解密程序的大致步骤。请注意,这是一个简化的版本,实际应用中需要处理更多的边缘情况和错误检查。
首先,你需要包括必要的头文件,并声明一些常量如模数、公钥和私钥:
```cpp
#include <iostream>
#include <vector>
const long long MOD = 1000000007;
std::vector<long long> publicKey(2), privateKey(2);
```
然后定义生成公钥和私钥的过程:
```cpp
void generateKeys() {
// ... 使用大素数生成算法生成两个大质数 p 和 q
// 计算 n = p * q 和 totient(n) = (p - 1) * (q - 1)
// 分配 p-1 和 q-1 给 e 和 d,满足 e * d ≡ 1 (mod totient(n))
// 公钥为 (n, e),私钥为 (n, d)
}
```
接下来是加密函数,这里采用模指数运算:
```cpp
std::string encrypt(const std::string& message) {
std::vector<long long> encryptedMessage(message.length());
for (size_t i = 0; i < message.size(); ++i) {
encryptedMessage[i] = pow(message[i], publicKey[0]) % MOD;
}
return encryptedMessage;
}
```
对于解密,也使用同样的模指数运算,但需要用到私钥:
```cpp
std::string decrypt(const std::vector<long long>& ciphertext) {
std::string decryptedMessage(ciphertext.size(), ' ');
for (size_t i = 0; i < ciphertext.size(); ++i) {
decryptedMessage[i] = pow(ciphertext[i], privateKey[0]) % MOD;
}
return decryptedMessage;
}
```
最后,主函数用于演示加密和解密过程:
```cpp
int main() {
generateKeys();
// ... 接收输入并进行加密,存储到encryptedVector
std::string decryptedMessage = decrypt(encryptedVector);
// ... 输出解密后的消息
return 0;
}
```
阅读全文
相关推荐
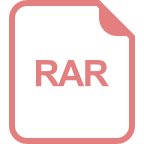
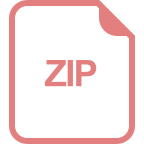















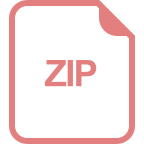