用c++语言写rsa加密算法
时间: 2023-08-04 16:38:59 浏览: 62
以下是使用C++语言实现RSA加密算法的示例代码:
```c++
#include <iostream>
#include <cmath>
#include <string>
using namespace std;
// 求最大公约数
int gcd(int a, int b) {
if (b == 0) {
return a;
}
return gcd(b, a % b);
}
// 判断是否为质数
bool isPrime(int num) {
if (num < 2) {
return false;
}
for (int i = 2; i <= sqrt(num); i++) {
if (num % i == 0) {
return false;
}
}
return true;
}
// 生成RSA密钥
void generateRSAKey(int p, int q, int &n, int &e, int &d) {
n = p * q; // 计算n
int phi = (p - 1) * (q - 1); // 计算欧拉函数phi(n)
do {
e = rand() % (phi - 2) + 2; // 随机生成一个整数e,2 <= e <= phi(n) - 1
} while (gcd(e, phi) != 1); // e和phi(n)要互质
int k = 1;
while ((k * phi + 1) % e != 0) { // 计算d,使得 (k * phi + 1) % e = 0
k++;
}
d = (k * phi + 1) / e; // 计算d
}
// RSA加密
string rsaEncrypt(string plainText, int n, int e) {
string cipherText = "";
for (int i = 0; i < plainText.size(); i++) {
int m = plainText[i]; // 明文转换为ASCII码
int c = (int)pow(m, e) % n; // 计算密文
cipherText += to_string(c) + " ";
}
return cipherText;
}
// RSA解密
string rsaDecrypt(string cipherText, int n, int d) {
string plainText = "";
string str = "";
for (int i = 0; i < cipherText.size(); i++) {
if (cipherText[i] != ' ') {
str += cipherText[i];
} else {
int c = stoi(str); // 将字符串转换为整数
int m = (int)pow(c, d) % n; // 计算明文
plainText += (char)m; // 将ASCII码转换为字符
str = "";
}
}
return plainText;
}
int main() {
int p = 61; // 选取两个质数p和q
int q = 53;
int n, e, d;
generateRSAKey(p, q, n, e, d); // 生成RSA密钥
string plainText = "Hello, world!"; // 明文
string cipherText = rsaEncrypt(plainText, n, e); // RSA加密
string decryptedText = rsaDecrypt(cipherText, n, d); // RSA解密
cout << "明文:" << plainText << endl;
cout << "密文:" << cipherText << endl;
cout << "解密后的明文:" << decryptedText << endl;
return 0;
}
```
这段代码实现了RSA加密和解密功能,其中generateRSAKey函数用于生成RSA密钥,rsaEncrypt函数用于加密明文,rsaDecrypt函数用于解密密文。
相关推荐
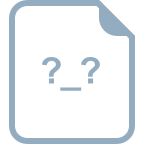
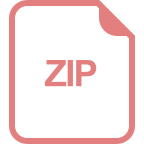














