用c++写一个RSA加密算法
时间: 2023-07-01 10:23:58 浏览: 45
好的,以下是一个简单的RSA加密算法的C++实现:
```cpp
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <cmath>
using namespace std;
// 判断一个数是否为素数
bool is_prime(int n) {
if (n <= 1) return false;
for (int i = 2; i <= sqrt(n); i++) {
if (n % i == 0) return false;
}
return true;
}
// 生成大素数p和q
void generate_pq(int &p, int &q) {
srand((unsigned)time(NULL));
while (true) {
p = rand() % 100 + 100;
if (is_prime(p)) break;
}
while (true) {
q = rand() % 100 + 100;
if (is_prime(q) && q != p) break;
}
}
// 计算a^b mod p
int mod_pow(int a, int b, int p) {
int res = 1;
while (b > 0) {
if (b & 1) res = res * a % p;
b >>= 1;
a = a * a % p;
}
return res;
}
// 计算两个数的最大公约数
int gcd(int a, int b) {
if (a < b) swap(a, b);
if (b == 0) return a;
return gcd(b, a % b);
}
// 计算e的逆元
int inverse(int e, int phi) {
int x1 = 1, x2 = 0, x3 = phi;
int y1 = 0, y2 = 1, y3 = e;
int q, t1, t2, t3;
while (y3 != 1) {
q = x3 / y3;
t1 = x1 - q * y1; t2 = x2 - q * y2; t3 = x3 - q * y3;
x1 = y1; x2 = y2; x3 = y3;
y1 = t1; y2 = t2; y3 = t3;
}
return y2 > 0 ? y2 : y2 + phi;
}
// 生成公钥和私钥
void generate_key(int &n, int &e, int &d) {
int p, q;
generate_pq(p, q);
n = p * q;
int phi = (p - 1) * (q - 1);
while (true) {
e = rand() % (phi - 2) + 2;
if (gcd(e, phi) == 1) break;
}
d = inverse(e, phi);
}
// 加密
int encrypt(int message, int n, int e) {
return mod_pow(message, e, n);
}
// 解密
int decrypt(int cipher, int n, int d) {
return mod_pow(cipher, d, n);
}
int main() {
int n, e, d;
int message = 12345;
generate_key(n, e, d);
int cipher = encrypt(message, n, e);
int decrypted = decrypt(cipher, n, d);
cout << "n = " << n << endl;
cout << "e = " << e << endl;
cout << "d = " << d << endl;
cout << "message = " << message << endl;
cout << "cipher = " << cipher << endl;
cout << "decrypted = " << decrypted << endl;
return 0;
}
```
以上代码实现了RSA加密算法,并且可以生成随机的公钥和私钥,并用公钥进行加密和私钥进行解密。
相关推荐
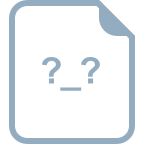














