for iteration in range(it_time): wx = torch.mm(train_x, w) # 前向传播 loss = (0.5 * (train_y - wx) ** 2).mean() # 计算 MSE loss loss.backward() # 反向传播 y_train_loss[iteration] = loss w.data.sub_(lr*w.grad) # w = w - lr*w.grad w.grad.zero_() # w的梯度清零,如果不清零,会一直累加 if iteration%100 == 0 and iteration!=0: wx_val = torch.mm(val_x, w) y_val_loss[int(iteration/100)] = (0.5 * (val_y - wx_val) ** 2).mean() 怎么保存这段代码的权重信息
时间: 2024-02-15 08:20:03 浏览: 22
要保存这段代码的权重信息,你可以使用 `torch.save()` 方法将模型的参数保存到文件中。在你的代码中,你可以在循环结束后添加以下代码来保存权重信息:
```python
torch.save(w, 'model_weights.pth')
```
这会将变量 `w` 的权重保存到名为 `model_weights.pth` 的文件中。你可以自定义文件名和路径。
如果你想要保存整个模型,包括其他参数和网络结构等信息,可以使用以下代码:
```python
torch.save(model, 'model.pth')
```
其中,`model` 是你的模型对象。这样会将整个模型保存到名为 `model.pth` 的文件中。
要加载已保存的权重信息,可以使用 `torch.load()` 方法。例如,要加载之前保存的 `model_weights.pth` 文件中的权重,可以使用以下代码:
```python
w = torch.load('model_weights.pth')
```
这会将权重加载到变量 `w` 中。如果要加载整个模型,可以使用以下代码:
```python
model = torch.load('model.pth')
```
请注意,加载模型时,你需要确保与保存时的模型结构和参数匹配。
相关问题
def FGSM(self, x, y_true, y_target=None, eps=0.03, alpha=2/255, iteration=1): self.set_mode('eval') x = Variable(cuda(x, self.cuda), requires_grad=True) y_true = Variable(cuda(y_true, self.cuda), requires_grad=False) if y_target is not None: targeted = True y_target = Variable(cuda(y_target, self.cuda), requires_grad=False) else: targeted = False h = self.net(x) prediction = h.max(1)[1] accuracy = torch.eq(prediction, y_true).float().mean() cost = F.cross_entropy(h, y_true) if iteration == 1: if targeted: x_adv, h_adv, h = self.attack.fgsm(x, y_target, True, eps) else: x_adv, h_adv, h = self.attack.fgsm(x, y_true, False, eps) else: if targeted: x_adv, h_adv, h = self.attack.i_fgsm(x, y_target, True, eps, alpha, iteration) else: x_adv, h_adv, h = self.attack.i_fgsm(x, y_true, False, eps, alpha, iteration) prediction_adv = h_adv.max(1)[1] accuracy_adv = torch.eq(prediction_adv, y_true).float().mean() cost_adv = F.cross_entropy(h_adv, y_true) # make indication of perturbed images that changed predictions of the classifier if targeted: changed = torch.eq(y_target, prediction_adv) else: changed = torch.eq(prediction, prediction_adv) changed = torch.eq(changed, 0) changed = changed.float().view(-1, 1, 1, 1).repeat(1, 3, 28, 28) changed[:, 0, :, :] = where(changed[:, 0, :, :] == 1, 252, 91) changed[:, 1, :, :] = where(changed[:, 1, :, :] == 1, 39, 252) changed[:, 2, :, :] = where(changed[:, 2, :, :] == 1, 25, 25) changed = self.scale(changed/255) changed[:, :, 3:-2, 3:-2] = x_adv.repeat(1, 3, 1, 1)[:, :, 3:-2, 3:-2] self.set_mode('train') return x_adv.data, changed.data,\ (accuracy.item(), cost.item(), accuracy_adv.item(), cost_adv.item())
这段代码是一个实现了FGSM(Fast Gradient Sign Method)攻击的函数。FGSM是一种用于生成对抗样本的方法,通过在输入样本上添加一小步扰动来欺骗分类器,使其产生错误的分类结果。
该函数的输入参数包括原始输入样本x、真实标签y_true、目标标签y_target(可选)、扰动范围eps、扰动步长alpha和迭代次数iteration。
函数首先将模型设置为评估模式,然后将输入样本转化为可求导变量,并计算原始样本在模型上的预测结果和准确率。然后计算原始样本在模型上的交叉熵损失。
接下来根据迭代次数选择使用FGSM攻击还是I-FGSM(Iterative FGSM)攻击。如果目标标签y_target存在,则使用目标攻击,否则使用非目标攻击。攻击过程中,对输入样本添加扰动,并计算扰动后的样本在模型上的预测结果和准确率。
最后,将产生的对抗样本、扰动图像、原始样本在模型上的准确率和损失作为输出返回。
值得注意的是,代码中还包含了一个用于生成扰动图像的部分。首先根据模型预测结果确定哪些样本的分类结果发生了变化,然后将这些样本的对应像素位置修改为特定的颜色值,以突出显示扰动部分。最后将扰动图像与对抗样本叠加,形成最终的扰动图像。
请问我还能为您解答其他问题吗?
根据前端传过来的前端传递过来的开始时间和结束时间参数 筛选创建时间在开始时间和结束时间区间的数据 修改后端写法def get_bugs_data(): api_url = "https://api.tapd.cn/bugs" api_headers = {"Authorization": "#", "content-type": "application/json"}iterations_url = "https:/"count_url ="https://at" params_count = {"status": "closed","workspace_id": 41571821, } # 发送请求,获取缺陷总数 response = requests.get(count_url, params=params_count, headers=api_headers) if response.status_code == 200: total_bug = response.json()total_count = total_bug['data']['count'] print(total_count) else: print("Error: Failed to retrieve count. Status code: ", response.status_code) start_time = request.get('start_time') end_time = request.get('end_time') start_time =datetime.strptime(start_time, '%Y-%m-%d %H:%M:%S') end_time = datetime.strptime(end_time, '%Y-%m-%d %H:%M:%S') bug_list = [] for i in range(1,total_count//200): params = {"limit": 200, "status": "closed", "workspace_id": 41571821, "page": i } response = requests.get(api_url, params=params, headers=api_headers) if response.status_code == 200: res = response.json() for bug_item in res['data']: if bug_item['Bug']['fixer'] and bug_item['Bug']['fixer'] in white_name:bug_data_dict = {"bug_title": bug_item['Bug']['title'], "bug_created": bug_item['Bug']['created'], "bug_fixer": bug_item['Bug']['fixer'], "bug_resolved": bug_item['Bug']['resolved'], "bug_url": f"https://www.tapd.cn/41571821/bugtrace/bugs/view?bug_id={bug_item['Bug']['id']}" } params_iteration = {"limit": 200, "workspace_id": 41571821, "id": bug_item['Bug']['iteration_id'] }response =requests.get(iterations_url, params=params_iteration, headers=api_headers)if response.status_code == 200: iterations = response.json() iteration_name = iterations['data'][0]['Iteration']['name'] bug_data_dict['iteration_name'] = iteration_name bug_list.append(bug_data_dict) else: print("Error: Failed to retrieve iterations. Status code: ", response.status_code) return {"code": 200, "msg": "成功", "data": bug_list}
可以将代码中的bug数据筛选部分修改如下:
```python
bug_list = []
for i in range(1, total_count//200 + 2):
params = {"limit": 200, "status": "closed", "workspace_id": 41571821, "page": i}
response = requests.get(api_url, params=params, headers=api_headers)
if response.status_code == 200:
res = response.json()
for bug_item in res['data']:
created_time = datetime.strptime(bug_item['Bug']['created'], '%Y-%m-%d %H:%M:%S')
if start_time <= created_time <= end_time:
if bug_item['Bug']['fixer'] and bug_item['Bug']['fixer'] in white_name:
bug_data_dict = {"bug_title": bug_item['Bug']['title'],
"bug_created": bug_item['Bug']['created'],
"bug_fixer": bug_item['Bug']['fixer'],
"bug_resolved": bug_item['Bug']['resolved'],
"bug_url": f"https://www.tapd.cn/41571821/bugtrace/bugs/view?bug_id={bug_item['Bug']['id']}"}
params_iteration = {"limit": 200, "workspace_id": 41571821, "id": bug_item['Bug']['iteration_id']}
response = requests.get(iterations_url, params=params_iteration, headers=api_headers)
if response.status_code == 200:
iterations = response.json()
iteration_name = iterations['data'][0]['Iteration']['name']
bug_data_dict['iteration_name'] = iteration_name
bug_list.append(bug_data_dict)
else:
print("Error: Failed to retrieve iterations. Status code: ", response.status_code)
else:
print("Error: Failed to retrieve bugs. Status code: ", response.status_code)
return {"code": 200, "msg": "成功", "data": bug_list}
```
在每个缺陷被处理时,将其创建时间转换为datetime对象,然后与开始时间和结束时间进行比较。如果创建时间在时间范围内,则将该缺陷加入到结果列表中。
相关推荐
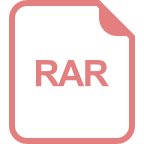
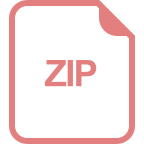
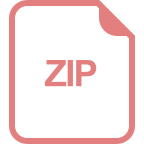













