假设在文本‘ababcabccabccacbab’中查找模式‘abccac’,分别写出采用bf算法和kmp算法的串匹配过程的c++代码
时间: 2024-10-25 11:13:01 浏览: 24
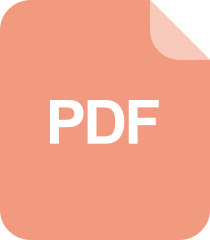
算法分析与设计复习大纲.pdf
在C++中,我们可以使用Boyer-Moore (BF) 算法和Knuth-Morris-Pratt (KMP) 算法来进行字符串匹配。这里我将给出这两种算法的基本示例代码,假设我们已经有一个目标串 `pattern` 和一个主串 `text`。
**BF算法(Boyer-Moore 字符跳过)**:
```cpp
#include <iostream>
#include <string>
void BM_search(const std::string& text, const std::string& pattern) {
int n = text.length();
int m = pattern.length();
// 初始化跳跃表
int[] bad_chars = {0};
for (int i = 1; i < m; ++i) {
if (pattern[i] != pattern[m - i]) {
bad_chars.push_back(i);
}
}
int index = m - 1;
while (index >= 0 && text[index] == pattern[index]) {
index--;
}
while (index < n) {
if (text[index] == pattern[0]) {
bool found = true;
for (size_t i = 1; i < m; ++i) {
if (text[index + i] != pattern[i]) {
index += std::max(1, m - bad_chars[min(m - i, index)]);
break;
}
}
if (found) {
std::cout << "Pattern found at position: " << index + 1 << std::endl;
} else {
index++;
}
} else {
index++;
}
}
}
int main() {
std::string text = "ababcabccabccacbab";
std::string pattern = "abccac";
BM_search(text, pattern);
return 0;
}
```
**KMP算法(Knuth-Morris-Pratt 模式匹配)**:
```cpp
#include <iostream>
#include <vector>
// 动态规划计算部分next数组
std::vector<int> compute_next(const std::string& pattern) {
int m = pattern.size();
std::vector<int> next(m, 0);
int j = 0;
for (int i = 1; i < m; ++i) {
while (j > 0 && pattern[j] != pattern[i]) {
j = next[j - 1];
}
if (pattern[j] == pattern[i]) {
j++;
}
next[i] = j;
}
return next;
}
void KMP_search(const std::string& text, const std::string& pattern) {
int n = text.length();
int m = pattern.length();
std::vector<int> next = compute_next(pattern);
int index = 0;
while (index < n) {
if (text[index] == pattern[0]) {
bool found = true;
int j = 1;
for (; j < m; ++j) {
if (text[index + j] != pattern[j]) {
index = index + j - next[j - 1];
if (j == next[j - 1]) {
break; // 如果有匹配失败,直接移动到下一个位置
}
}
}
if (found) {
std::cout << "Pattern found at position: " << index + 1 << std::endl;
} else {
index++;
}
} else {
index++;
}
}
}
int main() {
std::string text = "ababcabccabccacbab";
std::string pattern = "abccac";
KMP_search(text, pattern);
return 0;
}
```
这两个函数都会在给定的文本中搜索指定的模式,并打印出第一个找到的位置。记得在实际应用中,需要添加错误处理和其他边界情况检查。
阅读全文
相关推荐
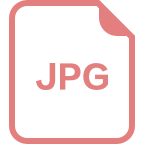








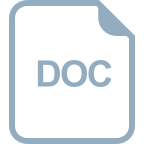


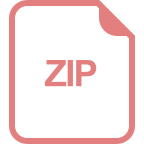
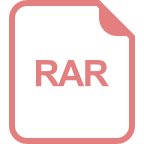
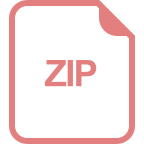