假设在文本‘ababcabccabccacbab’中查找模式‘abccac’,写出采用bf算法的串匹配过程的c++代码
时间: 2024-10-26 14:02:44 浏览: 46
在C++中,我们可以使用KMP(Knuth-Morris-Pratt)算法,它是Boyer-Moore的一种改进版本,用于字符串匹配,特别是当模式长度比主字符串短时效率更高。BF(Brute Force)是一种简单暴力的匹配方法,而KMP算法适合于有预处理的场景。下面是使用BF算法进行字符串匹配的过程,假设我们还没有KMP算法:
```cpp
#include <iostream>
#include <string>
std::string pattern = "abccac";
std::string text = "ababcabccabccacbab";
// BF搜索函数
int bruteForceMatch() {
for (size_t i = 0; i <= text.size() - pattern.size(); ++i) {
bool matchFound = true;
for (size_t j = 0; j < pattern.size(); ++j) {
if (text[i + j] != pattern[j]) {
matchFound = false;
break;
}
}
if (matchFound) {
return i;
}
}
return -1; // 如果未找到匹配,则返回-1表示失败
}
int main() {
int result = bruteForceMatch();
if (result == -1) {
std::cout << "Pattern not found in the text.\n";
} else {
std::cout << "Pattern starts at index: " << result << "\n";
}
return 0;
}
```
在这个例子中,`bruteForceMatch()`函数遍历主字符串`text`的所有可能起始位置,逐字符对比模式`pattern`,直到找到完全匹配的部分。
阅读全文
相关推荐
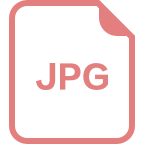















