C语言二叉树递归算法的实例代码实现
时间: 2024-12-08 22:13:26 浏览: 27
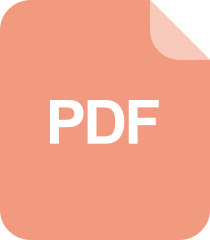
C语言二叉树的非递归遍历实例分析

在C语言中,二叉树的递归算法通常涉及创建节点、插入节点以及遍历操作。这里是一个简单的二叉搜索树(BST)插入节点的递归示例:
```c
#include <stdio.h>
// 定义二叉树节点结构体
typedef struct Node {
int data;
struct Node* left;
struct Node* right;
} Node;
// 插入新节点到BST
Node* insert(Node* root, int value) {
// 如果根为空,创建新节点作为根
if (root == NULL) {
return (Node*)malloc(sizeof(Node));
root = new Node;
root->data = value;
root->left = NULL;
root->right = NULL;
}
// 否则,比较值并递归处理左右子树
else if (value < root->data)
root->left = insert(root->left, value);
else
root->right = insert(root->right, value);
return root;
}
// 用于演示的简单主函数
int main() {
Node* root = NULL;
root = insert(root, 50); // 插入50
insert(root, 30); // 插入30
insert(root, 70); // 插入70
// ...其他节点
// 更深入地操作树...
// 示例代码不完整,仅展示了基本框架
return 0;
}
```
在这个例子中,`insert` 函数通过递归实现了对二叉搜索树的插入操作。当遇到空节点时,就创建一个新的节点;否则,根据节点值的大小选择左或右子树继续递归。
阅读全文
相关推荐
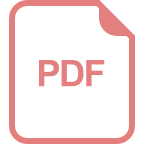
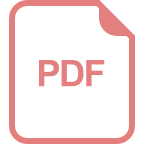
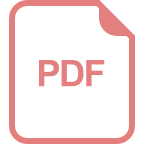
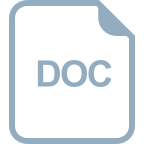
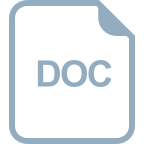
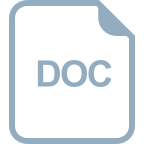
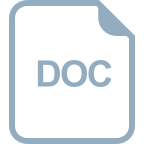
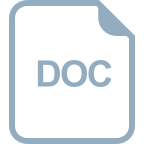
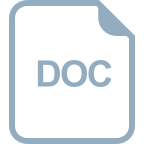
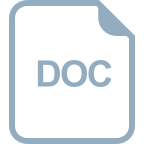
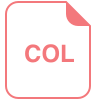
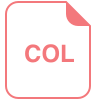



