反距离加权算法C++
时间: 2024-01-05 07:04:00 浏览: 27
反距离加权插值算法是一种常用的插值方法,它根据待插值点与样本点之间的距离来决定样本点对待插值点的影响权重。距离越近的样本点对待插值点的影响越大,距离越远的样本点对待插值点的影响越小。
以下是一个使用C++实现反距离加权插值算法的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <cmath>
// 定义样本点的结构体
struct SamplePoint {
double x;
double y;
double value;
};
// 计算两点之间的欧氏距离
double calculateDistance(double x1, double y1, double x2, double y2) {
return std::sqrt(std::pow(x2 - x1, 2) + std::pow(y2 - y1, 2));
}
// 反距离加权插值算法
double inverseDistanceWeighting(std::vector<SamplePoint>& samplePoints, double x, double y, double power) {
double numerator = 0.0;
double denominator = 0.0;
for (const auto& point : samplePoints) {
double distance = calculateDistance(x, y, point.x, point.y);
double weight = std::pow(1 / distance, power);
numerator += weight * point.value;
denominator += weight;
}
return numerator / denominator;
}
int main() {
// 样本点数据
std::vector<SamplePoint> samplePoints = {
{1.0, 2.0, 3.0},
{3.0, 4.0, 5.0},
{5.0, 6.0, 7.0}
};
// 待插值点坐标
double x = 2.5;
double y = 3.5;
// 反距离加权插值
double interpolatedValue = inverseDistanceWeighting(samplePoints, x, y, 2);
std::cout << "Interpolated value: " << interpolatedValue << std::endl;
return 0;
}
```
该示例代码中,首先定义了一个样本点的结构体`SamplePoint`,包含了样本点的坐标和值。然后定义了一个计算两点之间欧氏距离的函数`calculateDistance`。接下来是反距离加权插值算法`inverseDistanceWeighting`,它根据样本点的坐标、值和待插值点的坐标,计算出待插值点的插值结果。最后,在`main`函数中定义了样本点数据,以及待插值点的坐标,调用`inverseDistanceWeighting`函数进行插值,并输出插值结果。
相关推荐
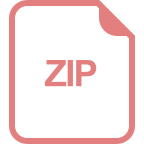
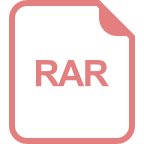














