vue create async await 使用
时间: 2023-08-21 09:12:14 浏览: 159
在Vue中使用async/await可以帮助我们更方便地处理异步操作。首先,我们需要在函数前面加上async关键字来声明这个函数是异步的。然后,我们可以使用await关键字来等待一个异步方法执行完成。
例如,我们可以创建一个async函数来处理异步操作:
```javascript
async function fetchData() {
const response = await axios.get('/api/data'); // 使用await等待异步请求完成
const data = response.data;
// 对获取到的数据进行处理
return data;
}
```
在Vue中,我们可以在组件的生命周期钩子函数中使用async/await来处理异步操作。例如,在created钩子函数中使用async/await来获取数据并进行渲染:
```javascript
export default {
async created() {
const data = await fetchData(); // 使用await等待数据获取完成
// 对获取到的数据进行渲染
}
}
```
需要注意的是,使用async/await时,我们需要将包含await的代码放在async函数中,以便正确处理异步操作的顺序。在上述示例中,await会阻塞后续表达式的执行,确保在获取数据完成之后再进行渲染。
希望以上信息对您有所帮助![1]
相关问题
```vue checkIn = createApp(CheckIn, { cardClose: () => { console.log(111111); checkIn && checkIn.unmount() document.body.removeChild(containerEle as HTMLElement) }, checkInCallback: async () => { await queryTaskList() }, }) ``` 传递如上。方法传递不起作用
对于Vue.js应用中的方法传递问题,可以尝试以下几个步骤来解决:
1. 确保`CheckIn`组件和`createApp`方法正确导入,并且在当前作用域中可用。
2. 确保`createApp`方法的第一个参数是`CheckIn`组件的实例或者是一个Vue组件对象。
3. 确保`cardClose`和`checkInCallback`方法在`CheckIn`组件中正确定义和导出。
4. 确保`checkIn`对象已经正确创建,并且可以在需要的地方访问到。
如果以上步骤都没有问题,但方法传递仍然不起作用,可以尝试以下调试方法:
1. 在`cardClose`和`checkInCallback`方法中添加`console.log`语句,以确认它们是否被调用。
2. 检查浏览器的开发者工具控制台,查看是否有任何错误提示。
3. 检查其他可能与方法传递相关的代码,例如事件绑定或组件嵌套。
如果问题仍然存在,请提供更多关于你的代码和错误信息的详细信息,以便我能够更好地帮助你解决问题。
请帮我优化下面的代码,解决错误 代码是: <template> <component :is="dynamicComponent" /> </template> <script> import axios from 'axios' import { compile, createApp,defineAsyncComponent } from 'vue' export default { data() { return { dynamicComponent: { template: '<div>Loading...</div>' }, renderFunction: null } }, async mounted() { const componentCode = await this.fetchComponentCode() const component = await eval(componentCode) this.dynamicComponent = defineAsyncComponent(() => component) }, methods: { async fetchComponentCode() { let param = { table: 't_code', where: " and ky='vue-test' " }; this.axios .post('/base/crud/list', param) .then(response => { if (response.data.success) { debugger return response.data.data[0].code } }) .catch(error => { }) } }, render() { } } </script>
下面是优化后的代码:
```
<template>
<component :is="dynamicComponent" />
</template>
<script>
import axios from 'axios'
import { defineAsyncComponent, createApp } from 'vue'
export default {
data() {
return {
dynamicComponent: defineAsyncComponent(() => import('./Loading.vue')),
componentCode: null
}
},
async mounted() {
const componentCode = await this.fetchComponentCode()
this.componentCode = componentCode
const component = this.createDynamicComponent(componentCode)
this.dynamicComponent = defineAsyncComponent(() => component)
},
methods: {
async fetchComponentCode() {
let param = { table: 't_code', where: " and ky='vue-test' " }
try {
const response = await this.axios.post('/base/crud/list', param)
if (response.data.success) {
return response.data.data[0].code
}
} catch (error) {
console.error(error)
}
},
createDynamicComponent(code) {
const app = createApp({
template: code
})
return app.component('dynamic-component')
}
}
}
</script>
```
优化后的代码主要做了以下几个方面的改进:
1. 在 data 中初始化 dynamicComponent 为一个 Loading 组件,避免一开始没有取到组件码时页面空白。
2. 将动态组件的创建逻辑封装成 createDynamicComponent 方法,将组件码作为参数传入,这样方便后面的调用。
3. 将 fetchComponentCode 方法中的 Promise 改写成 async/await,使代码更加简洁易读。
4. 将 axios 的 catch 中的代码改写成 console.error,这样可以更好地捕获错误信息。
阅读全文
相关推荐
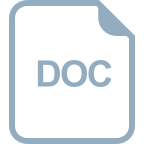
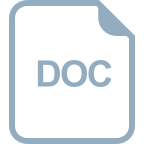
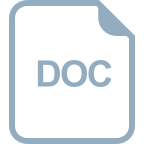
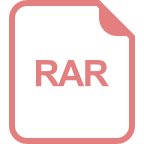
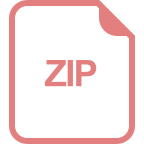
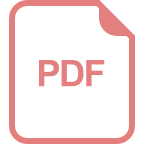










