怎么用c++ 写一个"几何体"基类
时间: 2024-10-08 16:03:49 浏览: 31
在C++中,你可以创建一个名为`Geometry`的基类来表示通用的几何体,它通常会包含一些基本属性(如位置、大小等)和通用的操作(如计算面积、体积)。这里是一个简单的例子:
```cpp
#include <iostream>
#include <cmath>
class Geometry {
protected:
double x, y; // 基本坐标
double size; // 尺寸
public:
// 构造函数
Geometry(double x, double y, double size) : x(x), y(y), size(size) {}
// 获取坐标和尺寸
double getX() const { return x; }
double getY() const { return y; }
double getSize() const { return size; }
// 计算面积或体积的基础方法(可能需要子类重写)
virtual double calculateArea() const = 0;
// 打印几何体信息
void display() const {
std::cout << "Position: (" << x << ", " << y << "), Size: " << size << std::endl;
}
};
// 子类示例:矩形和圆形
class Rectangle : public Geometry {
private:
double width;
public:
Rectangle(double x, double y, double size, double width) : Geometry(x, y, size), width(width) {}
double calculateArea() const override { return width * size; } // 重写父类方法
};
class Circle : public Geometry {
private:
double radius;
public:
Circle(double x, double y, double radius) : Geometry(x, y, radius * radius * M_PI), radius(radius) {} // 计算圆的半径影响面积
double calculateArea() const override { return M_PI * radius * radius; }
};
```
在这个例子中,`Geometry`是基类,`Rectangle`和`Circle`是它的派生类。它们都从`Geometry`继承了基本属性和`display()`方法,并覆盖了`calculateArea()`方法以适应各自的形状。
阅读全文
相关推荐
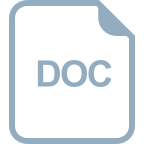
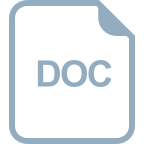
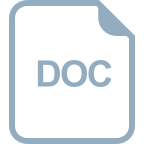
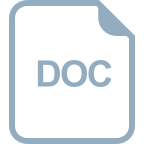



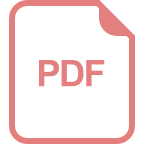
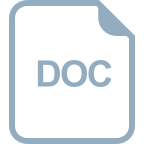
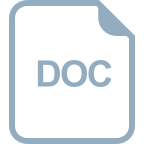
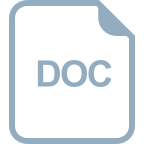
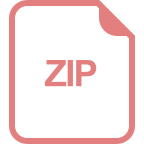
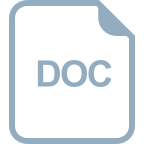
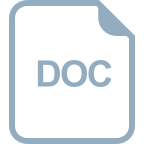
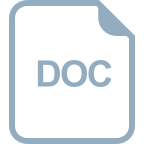



