如何使用Kahn算法实现字典序最小的拓扑排序,并通过伪代码和代码示例进行说明?
时间: 2024-11-16 14:20:52 浏览: 7
在有向无环图(DAG)中实现拓扑排序时,Kahn算法因其简洁性和高效性而受到青睐。要实现字典序最小的拓扑排序,我们需要在选择入度为0的节点加入排序结果列表时,优先选择编号最小的节点。以下是详细的实现步骤和伪代码,以及代码示例,帮助你理解和掌握这一过程:
参考资源链接:[Kahn算法与字典序最小拓扑排序:DAG的高效求解](https://wenku.csdn.net/doc/58qx7c5p1d?spm=1055.2569.3001.10343)
步骤1:初始化,统计每个节点的入度,并标记所有入度为0的节点。
步骤2:创建一个队列Q,并将所有入度为0的节点加入队列中。
步骤3:当队列Q不为空时,执行以下操作:
a. 取出队首元素,设为当前节点u。
b. 将当前节点u添加到拓扑排序的结果列表L中。
c. 遍历所有从u出发的边(u, v),将v的入度减1,并检查入度是否变为0,如果是,则将v加入队列Q中。
步骤4:若所有节点都被处理完毕,则算法成功,返回拓扑排序的结果列表L。若存在未处理节点,则图中存在环,无法进行拓扑排序。
伪代码如下:
```
function KahnTopologicalSort(graph):
inDegree = array of graph's vertices size filled with zeros
for each vertex in graph:
for each neighbor of vertex:
inDegree[neighbor]++
Q = empty queue
for each vertex in graph:
if inDegree[vertex] == 0:
Q.enqueue(vertex)
L = empty list
while not Q.isEmpty():
u = Q.dequeue()
L.append(u)
for each neighbor of u:
inDegree[neighbor]--
if inDegree[neighbor] == 0:
Q.enqueue(neighbor)
if size of L is not equal to the number of graph's vertices:
throw error indicating cycle in the graph
return L
```
代码示例(假设使用Python实现):
```python
def kahn_topological_sort(graph):
in_degree = [0] * len(graph)
queue = []
for i in range(len(graph)):
for v in graph[i]:
in_degree[v] += 1
for i in range(len(graph)):
if in_degree[i] == 0:
queue.append(i)
sorted_list = []
while queue:
u = queue.pop(0)
sorted_list.append(u)
for v in graph[u]:
in_degree[v] -= 1
if in_degree[v] == 0:
queue.append(v)
if len(sorted_list) != len(graph):
raise ValueError(
参考资源链接:[Kahn算法与字典序最小拓扑排序:DAG的高效求解](https://wenku.csdn.net/doc/58qx7c5p1d?spm=1055.2569.3001.10343)
阅读全文
相关推荐
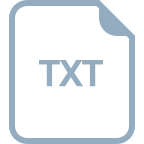
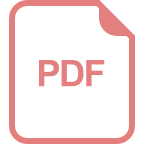
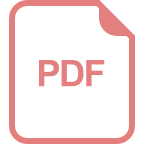















