如何在Python的Tkinter库中正确地插入和显示PNG图片?
时间: 2024-11-18 07:18:39 浏览: 9
在Python的Tkinter库中插入和显示PNG图片需要几个步骤:
1. 首先,确保你已经安装了`PIL`(Python Imaging Library)或者其更新版`Pillow`,这个库用于处理图像。你可以通过pip安装它:
```
pip install Pillow
```
2. 导入必要的模块:
```python
from tkinter import *
from PIL import Image, ImageTk
```
3. 加载PNG图片:
```python
img_path = 'path_to_your_image.png' # 替换为你图片的实际路径
image = Image.open(img_path)
```
4. 将图片转换为Tkinter可以接受的格式,这里通常使用`ImageTk.PhotoImage`:
```python
tk_img = ImageTk.PhotoImage(image)
```
5. 创建一个Tkinter的`Label`来显示图片,并设置其image属性:
```python
canvas = Canvas(root, width=image.width, height=image.height)
canvas.pack()
photo_label = Label(canvas, image=tk_img)
photo_label.image = tk_img # 给label保留一个引用,防止垃圾回收
photo_label.pack()
```
6. 最后,在主循环中运行Tkinter应用:
```python
root = Tk() # 创建主窗口
root.mainloop()
```
如果你想要动态加载或者更改图片,只需要改变`photo_label.config(image=tk_img)`中的`tk_img`即可。
阅读全文
相关推荐
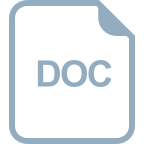
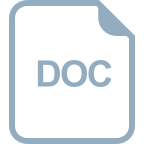
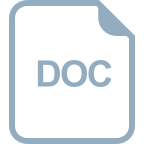
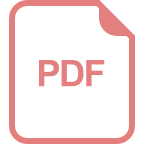
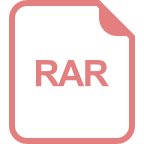
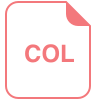
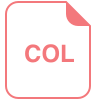
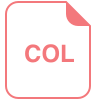
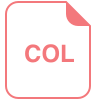
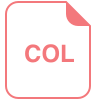
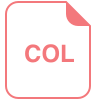
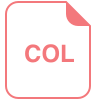
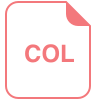
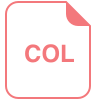
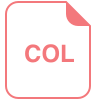
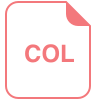
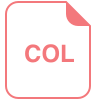
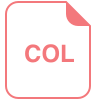
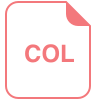