python构建齐次坐标变换矩阵,实现旋转,平移,缩放图像处理
时间: 2024-09-23 16:06:18 浏览: 97
在Python中,特别是通过NumPy库,我们可以方便地创建齐次坐标变换矩阵来处理图像的旋转、平移和缩放操作。齐次坐标是一种三维空间表示方式,它将二维点(x, y)扩展到(x, y, 1)的形式,便于几何变换的数学表达。
**1. 构建变换矩阵**
对于二维空间中的基本变换,我们有:
- **缩放**(Scale): 可以通过以下矩阵乘法完成,假设比例因子为s:
\[ S = \begin{bmatrix} s & 0 \\ 0 & s \end{bmatrix} \]
- **平移**(Translate): 通过添加偏移量向量(t_x, t_y)到每个点上,对应的矩阵是:
\[ T = \begin{bmatrix} 1 & 0 & t_x \\ 0 & 1 & t_y \\ 0 & 0 & 1 \end{bmatrix} \]
- **旋转**(Rotate): 使用旋转变换矩阵,如绕原点顺时针旋转θ角度,可以用`cv2.getRotationMatrix2D()`函数生成,然后应用`np.dot()`或`.dot()`方法:
\[ R(\theta) = \begin{bmatrix} \cos\theta & -\sin\theta & 0 \\ \sin\theta & \cos\theta & 0 \\ 0 & 0 & 1 \end{bmatrix} \]
组合这三个变换,可以得到总的变换矩阵 \( M = R(\theta) \cdot S \cdot T \)。
**示例代码**(使用OpenCV的transformations模块):
```python
import numpy as np
from cv2 import getRotationMatrix2D
def create_homogeneous_transform(rotate_degrees=0, scale=1, translate=(0, 0)):
# 计算旋转矩阵
rotate_rad = np.radians(rotate_degrees)
rotation_matrix = getRotationMatrix2D(center=(0, 0), angle=rotate_rad, scale=scale)
# 添加平移部分
translation_matrix = np.array([[1, 0, translate[0]], [0, 1, translate[1]], [0, 0, 1]])
# 组合变换矩阵
homogeneous_transform = np.dot(rotation_matrix, translation_matrix)
return homogeneous_transform
# 示例
homog_transform = create_homogeneous_transform(45, 2, (100, 50))
```
**
阅读全文
相关推荐
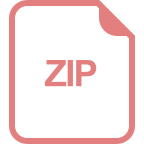
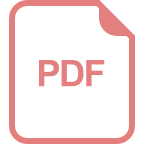
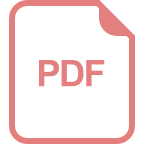
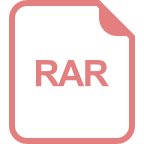
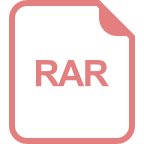
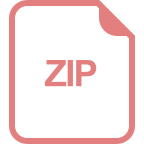
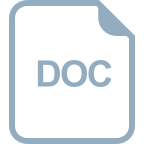
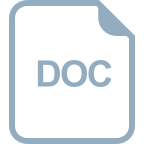
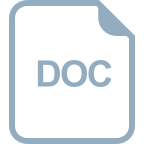
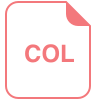
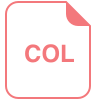
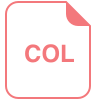
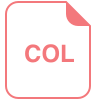






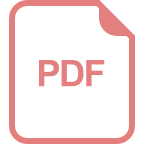