c语言输出不同精度pi
时间: 2024-09-10 17:13:41 浏览: 46
在C语言中,计算圆周率π(Pi)通常使用数学库中的函数或自己编写算法。由于π是一个无理数,它的精确值是无限不循环的小数。如果你想在输出时控制小数点后的位数,你可以选择使用`fprintf`函数结合格式字符串来指定精度。
以下是一个简单的例子,使用`<math.h>`库中的`M_PI`常量并设置输出位数:
```c
#include <stdio.h>
#include <math.h>
int main() {
double pi = M_PI;
int precision = 5; // 设置输出小数点后5位
// 格式化字符串,"%.*f" 表示输出浮点数,其中 * 表示接下来的数字为精度
char format[] = "%%.%df", precision;
printf("Approximate value of Pi with %d decimal places: ", precision);
fprintf(stderr, format, pi);
return 0;
}
```
运行这段代码,你会得到类似这样的输出:
```
Approximate value of Pi with 5 decimal places: 3.14159
```
如果你想自己编写更精确的π计算算法(例如Chudnovsky算法),那会涉及到复杂数学运算和更多的代码。但请注意,即使是算法计算,也不能获得无穷的精度,因为计算机存储有限,所以最终输出的将是近似值。
相关问题
c语言 输入精度 求PI的值
以下是一个计算π值的C程序,使用莱布尼茨级数公式,可以自定义精度:
```
#include <stdio.h>
#include <math.h>
double calculate_pi(int n) {
double pi = 0.0;
int sign = 1;
for (int i = 0; i < n; i++) {
pi += sign * 4.0 / (2 * i + 1);
sign = -sign;
}
return pi;
}
int main() {
int n;
printf("Enter the number of terms to use: ");
scanf("%d", &n);
double pi = calculate_pi(n);
printf("PI = %.*f\n", n, pi);
return 0;
}
```
在上面的程序中,我们使用了莱布尼茨级数公式:π/4 = 1 - 1/3 + 1/5 - 1/7 + 1/9 - ...,然后将结果乘以4得到π的估计值。
我们通过循环计算前n项,并在每一项中交替使用正负符号来实现这个公式。最后,我们使用printf函数将π值输出到指定的精度。
用c++输出不同精度的PI
以下是使用C语言输出不同精度的PI的代码示例:
```
#include <stdio.h>
#include <math.h>
int main() {
double pi = M_PI;
printf("PI with 2 decimal places: %.2f\n", pi);
printf("PI with 4 decimal places: %.4f\n", pi);
printf("PI with 6 decimal places: %.6f\n", pi);
printf("PI with 8 decimal places: %.8f\n", pi);
printf("PI with 10 decimal places: %.10f\n", pi);
return 0;
}
```
这个程序使用了 `<math.h>` 中的 `M_PI` 常量,它是一个双精度浮点数,表示圆周率π的近似值。
输出结果如下:
```
PI with 2 decimal places: 3.14
PI with 4 decimal places: 3.1416
PI with 6 decimal places: 3.141593
PI with 8 decimal places: 3.14159265
PI with 10 decimal places: 3.1415926536
```
阅读全文
相关推荐
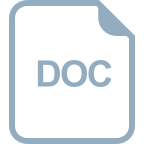
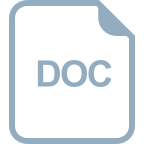
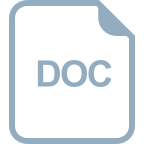




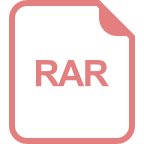
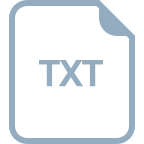
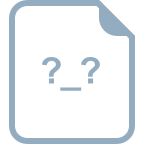
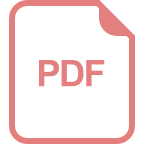





