pygame粒子效果
时间: 2024-02-05 19:03:55 浏览: 29
Pygame是一个用于开发2D游戏的Python库,它提供了丰富的功能和工具来创建游戏。粒子效果是游戏中常见的一种特效,可以用来模拟火焰、爆炸、烟雾等效果。
在Pygame中实现粒子效果可以通过以下步骤:
1. 创建粒子类:首先,你需要创建一个粒子类来表示每个粒子的属性和行为。这个类通常包含粒子的位置、速度、加速度等属性,并且有一个更新方法来更新粒子的状态。
2. 创建粒子系统类:接下来,你可以创建一个粒子系统类来管理所有的粒子。这个类通常包含一个粒子列表,以及添加、更新和绘制粒子的方法。
3. 更新粒子状态:在每一帧中,你需要更新每个粒子的状态,例如更新位置、速度等。你可以根据需要添加一些特效,例如重力、摩擦力等。
4. 绘制粒子:最后,你需要将每个粒子绘制到屏幕上。你可以使用Pygame提供的绘图函数来实现,例如使用圆形或者图片来表示粒子。
下面是一个简单的示例代码,展示了如何使用Pygame实现一个基本的粒子效果:
```python
import pygame
import random
# 粒子类
class Particle:
def __init__(self, pos):
self.pos = pos
self.vel = pygame.Vector2(random.uniform(-1, 1), random.uniform(-1, 1))
self.acc = pygame.Vector2(0, 0.1)
self.color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
def update(self):
self.vel += self.acc
self.pos += self.vel
def draw(self, screen):
pygame.draw.circle(screen, self.color, (int(self.pos.x), int(self.pos.y)), 5)
# 粒子系统类
class ParticleSystem:
def __init__(self):
self.particles = []
def add_particle(self, pos):
particle = Particle(pos)
self.particles.append(particle)
def update(self):
for particle in self.particles:
particle.update()
if particle.pos.y > 600:
self.particles.remove(particle)
def draw(self, screen):
for particle in self.particles:
particle.draw(screen)
# 初始化Pygame
pygame.init()
screen = pygame.display.set_mode((800, 600))
clock = pygame.time.Clock()
particle_system = ParticleSystem()
# 游戏主循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 添加粒子
mouse_pos = pygame.mouse.get_pos()
particle_system.add_particle(mouse_pos)
# 更新粒子系统
particle_system.update()
# 绘制粒子系统
screen.fill((0, 0, 0))
particle_system.draw(screen)
pygame.display.flip()
clock.tick(60)
pygame.quit()
```
这个示例代码实现了一个简单的粒子效果,每次点击鼠标左键会在鼠标位置添加一个粒子,并且粒子会受到重力的影响向下运动。你可以根据需要修改粒子的属性和行为,以及添加更多的特效来实现不同的粒子效果。
相关推荐
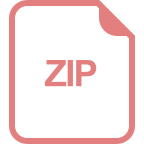














