基于深度学习的目标检测 代码
时间: 2023-09-01 21:07:29 浏览: 130
目标检测是计算机视觉中的一个重要问题,深度学习已经成为目标检测领域的主流方法之一。常见的深度学习目标检测算法包括 Faster R-CNN、YOLO 和 SSD 等。下面以 Faster R-CNN 为例介绍其目标检测的代码实现。
首先,需要安装 PyTorch 和 Torchvision:
```
pip install torch
pip install torchvision
```
然后,导入相关的库和模型:
```python
import torch
import torchvision
import cv2
import numpy as np
# 加载 Faster R-CNN 模型
model = torchvision.models.detection.fasterrcnn_resnet50_fpn(pretrained=True)
```
接下来,读取需要进行目标检测的图像:
```python
# 读取图像
img = cv2.imread('test.jpg')
```
为了输入到模型中,需要将图像转换为 PyTorch 的张量格式,并进行标准化:
```python
# 将图像转换为张量
img_tensor = torchvision.transforms.functional.to_tensor(img)
# 标准化图像
img_tensor = torchvision.transforms.functional.normalize(img_tensor, [0.485, 0.456, 0.406], [0.229, 0.224, 0.225])
```
接下来,将图像输入到模型中进行预测,并获取预测结果:
```python
# 将图像输入到模型中进行预测
model.eval()
with torch.no_grad():
prediction = model([img_tensor])
# 获取预测结果
boxes = prediction[0]['boxes'].cpu().numpy()
scores = prediction[0]['scores'].cpu().numpy()
labels = prediction[0]['labels'].cpu().numpy()
```
最后,将预测结果绘制在图像上:
```python
# 绘制预测框
for box, score, label in zip(boxes, scores, labels):
if score > 0.5:
box = box.astype(np.int32)
img = cv2.rectangle(img, (box[0], box[1]), (box[2], box[3]), (0, 255, 0), 2)
img = cv2.putText(img, f'{label}: {score:.2f}', (box[0], box[1]-5), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 1)
# 显示图像
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
完整的代码如下:
```python
import torch
import torchvision
import cv2
import numpy as np
# 加载 Faster R-CNN 模型
model = torchvision.models.detection.fasterrcnn_resnet50_fpn(pretrained=True)
# 读取图像
img = cv2.imread('test.jpg')
# 将图像转换为张量
img_tensor = torchvision.transforms.functional.to_tensor(img)
# 标准化图像
img_tensor = torchvision.transforms.functional.normalize(img_tensor, [0.485, 0.456, 0.406], [0.229, 0.224, 0.225])
# 将图像输入到模型中进行预测
model.eval()
with torch.no_grad():
prediction = model([img_tensor])
# 获取预测结果
boxes = prediction[0]['boxes'].cpu().numpy()
scores = prediction[0]['scores'].cpu().numpy()
labels = prediction[0]['labels'].cpu().numpy()
# 绘制预测框
for box, score, label in zip(boxes, scores, labels):
if score > 0.5:
box = box.astype(np.int32)
img = cv2.rectangle(img, (box[0], box[1]), (box[2], box[3]), (0, 255, 0), 2)
img = cv2.putText(img, f'{label}: {score:.2f}', (box[0], box[1]-5), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 1)
# 显示图像
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
注意:以上代码只是 Faster R-CNN 目标检测的示例,实际使用时需要根据具体的应用场景进行调整和优化。
阅读全文
相关推荐
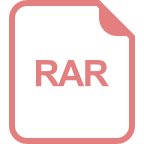
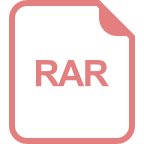
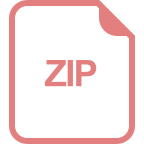

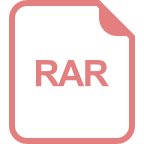
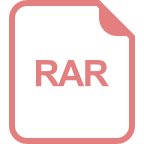
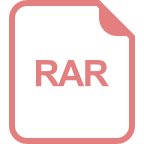
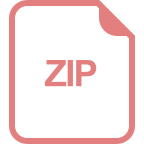
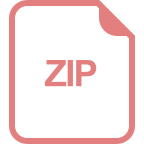
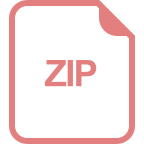
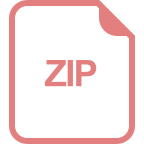


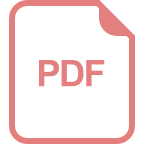
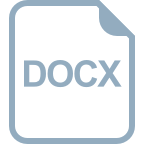