Python取出鸢尾花中的山鸢尾和维吉尼亚鸢尾
时间: 2024-03-06 17:42:34 浏览: 20
使用Python中的pandas库来读取数据集并进行筛选。具体步骤如下:
```python
import pandas as pd
# 读取数据集
data = pd.read_csv("iris.csv")
# 筛选出山鸢尾和维吉尼亚鸢尾
filtered_data = data[(data["Species"] == "setosa") | (data["Species"] == "virginica")]
# 输出筛选结果
print(filtered_data)
```
运行以上代码,即可取出鸢尾花中的山鸢尾和维吉尼亚鸢尾。
相关问题
tensorflow鸢尾花分类 山鸢尾和维吉尼亚鸢尾
以下是使用TensorFlow实现鸢尾花分类的代码,可以区分山鸢尾和维吉尼亚鸢尾:
```python
import tensorflow as tf
import pandas as pd
# 读取数据集
CSV_COLUMN_NAMES = ['SepalLength', 'SepalWidth', 'PetalLength', 'PetalWidth', 'Species']
SPECIES = ['Setosa', 'Versicolor', 'Virginica']
train_path = tf.keras.utils.get_file(
"iris_training.csv", "https://storage.googleapis.com/download.tensorflow.org/data/iris_training.csv")
test_path = tf.keras.utils.get_file(
"iris_test.csv", "https://storage.googleapis.com/download.tensorflow.org/data/iris_test.csv")
train = pd.read_csv(train_path, names=CSV_COLUMN_NAMES, header=0)
test = pd.read_csv(test_path, names=CSV_COLUMN_NAMES, header=0)
train_y = train.pop('Species')
test_y = test.pop('Species')
# 构建特征列
my_feature_columns = []
for key in train.keys():
my_feature_columns.append(tf.feature_column.numeric_column(key=key))
# 构建分类器
classifier = tf.estimator.DNNClassifier(
feature_columns=my_feature_columns,
hidden_units=[30, 10],
n_classes=3)
# 训练模型
train_input_fn = tf.compat.v1.estimator.inputs.pandas_input_fn(
x=train,
y=train_y,
batch_size=32,
num_epochs=None,
shuffle=True)
classifier.train(input_fn=train_input_fn, steps=2000)
# 评估模型
test_input_fn = tf.compat.v1.estimator.inputs.pandas_input_fn(
x=test,
y=test_y,
batch_size=32,
num_epochs=1,
shuffle=False)
eval_result = classifier.evaluate(input_fn=test_input_fn)
print('\nTest set accuracy: {accuracy:0.3f}\n'.format(**eval_result))
# 预测新数据
new_samples = pd.DataFrame({
'SepalLength': [5.1, 5.9, 6.9],
'SepalWidth': [3.3, 3.0, 3.1],
'PetalLength': [1.7, 4.2, 5.4],
'PetalWidth': [0.5, 1.5, 2.1],
})
predict_input_fn = tf.compat.v1.estimator.inputs.pandas_input_fn(
x=new_samples,
num_epochs=1,
shuffle=False)
predictions = list(classifier.predict(input_fn=predict_input_fn))
predicted_classes = [SPECIES[prediction['class_ids'][0]] for prediction in predictions]
print(
"New Samples, Class Predictions: {}\n"
.format(predicted_classes))
```
python bp 鸢尾花
Python BP 鸢尾花,指的是使用 Python 编程语言实现反向传播算法来训练神经网络,解决鸢尾花分类问题。
鸢尾花是一种常见的植物,有三个不同的品种:山鸢尾、变色鸢尾和维吉尼亚鸢尾。为了分类这三个品种,我们可以使用机器学习中的神经网络模型。
神经网络通常由多个层(输入层、隐藏层和输出层)组成,每一层都包含多个神经元,每个神经元都有一些权重和偏置。神经网络通过前向传播计算每个神经元的输出,并将这些输出传递到下一层,最终得到模型的输出。在反向传播算法中,我们利用训练数据中的真实标签与模型输出之间的差异,来调整神经元的权重和偏置,以使模型的输出更加接近真实标签,从而提高模型的准确性。
Python BP 鸢尾花的过程大致如下:首先,需要准备鸢尾花数据集;然后,我们可以使用 Python 中的 NumPy 库来构建神经网络结构,并初始化权重和偏置;接下来,我们可以通过反向传播算法来训练神经网络,不断调整权重和偏置,使得模型的预测结果与真实标签之间的误差越来越小;最后,我们可以使用训练好的模型来预测新的鸢尾花样本的品种。
Python BP 鸢尾花是一种经典的机器学习问题,也是许多初学者入门深度学习的第一个案例。通过学习这个案例,可以深入理解神经网络和反向传播算法的原理和应用,为后续的深度学习研究打下基础。
相关推荐
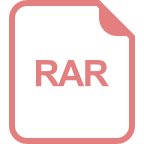
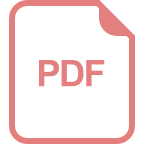
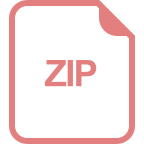












