大规模车辆路径问题算法及代码实现
时间: 2023-12-15 15:33:30 浏览: 35
大规模车辆路径问题是一个NP难问题,目前还没有找到一种能够在多项式时间内解决的算法。但是,有一些启发式算法可以用来近似求解该问题,例如遗传算法、模拟退火算法、禁忌搜索算法等。下面是一个使用遗传算法求解大规模车辆路径问题的Python代码示例:
```python
import random
# 车辆路径问题的遗传算法求解
class GA_TSP:
def __init__(self, city_num, pop_size, pc, pm, max_iter):
self.city_num = city_num # 城市数量
self.pop_size = pop_size # 种群大小
self.pc = pc # 交叉概率
self.pm = pm # 变异概率
self.max_iter = max_iter # 最大迭代次数
self.city_pos = [] # 城市坐标
self.pop = [] # 种群
self.fitness = [] # 适应度
self.best_path = [] # 最优路径
self.best_fitness = float('inf') # 最优适应度
# 初始化种群
def init_pop(self):
for i in range(self.pop_size):
path = list(range(self.city_num))
random.shuffle(path)
self.pop.append(path)
# 计算适应度
def calc_fitness(self, path):
dist = 0
for i in range(self.city_num - 1):
dist += ((self.city_pos[path[i]][0] - self.city_pos[path[i+1]][0])**2 + (self.city_pos[path[i]][1] - self.city_pos[path[i+1]][1])**2)**0.5
return 1 / dist
# 选择操作
def selection(self):
new_pop = []
for i in range(self.pop_size):
idx1, idx2 = random.sample(range(self.pop_size), 2)
if self.fitness[idx1] > self.fitness[idx2]:
new_pop.append(self.pop[idx1])
else:
new_pop.append(self.pop[idx2])
self.pop = new_pop
# 交叉操作
def crossover(self):
for i in range(0, self.pop_size, 2):
if random.random() < self.pc:
idx1, idx2 = random.sample(range(self.city_num), 2)
if idx1 > idx2:
idx1, idx2 = idx2, idx1
child1, child2 = [-1] * self.city_num, [-1] * self.city_num
for j in range(idx1, idx2+1):
child1[j] = self.pop[i+1][j]
child2[j] = self.pop[i][j]
idx = idx2 + 1
for j in range(self.city_num):
if self.pop[i][j] not in child1:
child1[idx] = self.pop[i][j]
idx = (idx + 1) % self.city_num
if self.pop[i+1][j] not in child2:
child2[idx] = self.pop[i+1][j]
idx = (idx + 1) % self.city_num
self.pop[i], self.pop[i+1] = child1, child2
# 变异操作
def mutation(self):
for i in range(self.pop_size):
if random.random() < self.pm:
idx1, idx2 = random.sample(range(self.city_num), 2)
self.pop[i][idx1], self.pop[i][idx2] = self.pop[i][idx2], self.pop[i][idx1]
# 迭代
def run(self):
self.init_pop()
for i in range(self.max_iter):
self.fitness = [self.calc_fitness(path) for path in self.pop]
best_idx = self.fitness.index(max(self.fitness))
if self.fitness[best_idx] > self.best_fitness:
self.best_path = self.pop[best_idx]
self.best_fitness = self.fitness[best_idx]
self.selection()
self.crossover()
self.mutation()
return self.best_path, self.best_fitness
if __name__ == '__main__':
city_num = 20 # 城市数量
pop_size = 100 # 种群大小
pc = 0.8 # 交叉概率
pm = 0.1 # 变异概率
max_iter = 1000 # 最大迭代次数
city_pos = [(random.random(), random.random()) for i in range(city_num)] # 随机生成城市坐标
ga_tsp = GA_TSP(city_num, pop_size, pc, pm, max_iter)
ga_tsp.city_pos = city_pos
best_path, best_fitness = ga_tsp.run()
print('最优路径:', best_path)
print('最优适应度:', best_fitness)
```
相关推荐
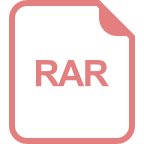
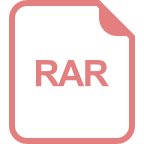












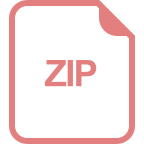
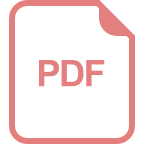
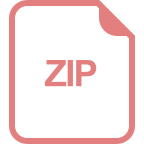