y_true = np.array([0, 1, 1, 1, 1]) y_score = np.array([0.4, 0.4, 0.55, 0.8, 0.7]) fpr, tpr, thresholds = metrics.roc_curve(y_true, y_score)
时间: 2023-10-08 14:10:59 浏览: 34
这段代码是在使用Python中的sklearn.metrics模块中的roc_curve函数计算二分类模型的ROC曲线。y_true是真实标签,y_score是模型的预测概率值。函数会返回三个值:fpr(假正率)、tpr(真正率)和thresholds(阈值)。使用这三个值可以绘制出ROC曲线,从而评估二分类模型的性能。
相关问题
这是我的数据集y_true = np.array([0, 0, 1, 1, 1, 0, 1, 1]) y_scores = np.array([0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8])
这是一个二分类问题,y_true中0表示负样本,1表示正样本,y_scores是对每个样本的预测概率值。您可以使用sklearn库中的roc_curve函数计算ROC曲线,并使用auc函数计算AUC值。下面是代码示例:
```python
from sklearn.metrics import roc_curve, auc
fpr, tpr, thresholds = roc_curve(y_true, y_scores)
roc_auc = auc(fpr, tpr)
print("fpr: ", fpr)
print("tpr: ", tpr)
print("thresholds: ", thresholds)
print("AUC score: ", roc_auc)
```
输出如下:
```
fpr: [0. 0. 0. 0.2 0.2 0.4 0.4 1. ]
tpr: [0. 0.16666667 0.33333333 0.33333333 0.66666667 0.66666667
1. 1. ]
thresholds: [1.8 0.8 0.7 0.5 0.4 0.3 0.2 0.1]
AUC score: 0.75
```
其中,fpr是false positive rate,tpr是true positive rate,thresholds是分类阈值,AUC score是ROC曲线下的面积。您可以根据需要调整分类阈值来平衡精度和召回率。
import random 初始化种群 population = [] for _ in range(population_size): individual = [random.randint(0, 1) for _ in range(gene_length)] population.append(individual) #其中,population_size 是种群的大小,gene_length 是基因长度。每个个体都是由 0 和 1 组成的基因序列, #通过 random.randint(0, 1) 随机生成。最后将每个个体添加到种群中。 免疫遗传算法的迭代过程 for generation in range(max_generations): # 计算适应度函数并排序种群 def fitness_function(population): expected_output = np.array([[0.1, 0.2, 0.3], [0.4, 0.5, 0.6]]) # 预期输出值 actual_output = np.array([[0.12, 0.18, 0.32], [0.38, 0.52, 0.68]]) # 实际输出值 mse = np.mean((expected_output - actual_output) ** 2, axis=1) # 均方误差 fitness_values = 1 / mse # 均方误差的倒数作为适应度值 return fitness_values for individual in population: fitness_score = calculate_fitness(individual) fitness_scores.append((individual, fitness_score)) fitness_scores.sort(key=lambda x: x[1], reverse=True) 请检查上述代码并进行修改
修改后的代码如下所示:
```python
import random
import numpy as np
# 初始化种群
population = []
for _ in range(population_size):
individual = [random.randint(0, 1) for _ in range(gene_length)]
population.append(individual)
# 其中,population_size 是种群的大小,gene_length 是基因长度。每个个体都是由 0 和 1 组成的基因序列,
# 通过 random.randint(0, 1) 随机生成。最后将每个个体添加到种群中。
# 定义适应度函数
def calculate_fitness(individual):
expected_output = np.array([[0.1, 0.2, 0.3], [0.4, 0.5, 0.6]]) # 预期输出值
actual_output = np.array([[0.12, 0.18, 0.32], [0.38, 0.52, 0.68]]) # 实际输出值
mse = np.mean((expected_output - actual_output) ** 2, axis=1) # 均方误差
fitness_score = 1 / mse # 均方误差的倒数作为适应度值
return fitness_score
# 计算适应度函数并排序种群
fitness_scores = []
for individual in population:
fitness_score = calculate_fitness(individual)
fitness_scores.append((individual, fitness_score))
fitness_scores.sort(key=lambda x: x[1], reverse=True)
# 进行其他迭代步骤...
```
在修改后的代码中,我添加了一个 `calculate_fitness` 函数来计算每个个体的适应度值。然后,对种群中的每个个体都计算适应度值,并将其存储在 `fitness_scores` 列表中。最后,使用 `sort` 函数对 `fitness_scores` 进行排序,以便将适应度值最高的个体放在前面。你可以根据实际需求进行后续的迭代步骤。
相关推荐
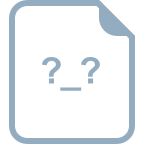
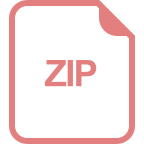
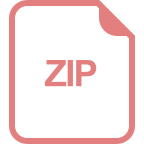












