Qt代码实现xml的增删改查,用函数实现。增加节点<该父节点下新增tagName名为variant的QDomElment>,删除节点<删除当前节点即所有子节点>,编辑该节点<修改节点属性的value值>
时间: 2024-02-21 12:01:38 浏览: 76
好的,下面是一个基于Qt的实现xml的增删改查的示例代码,其中包含了新增节点、删除节点和编辑节点的函数实现:
```cpp
#include <QFile>
#include <QDomDocument>
#include <QDomElement>
#include <QDebug>
// 读取xml文件并返回根节点
QDomElement readXmlFile(const QString& fileName)
{
QDomDocument xml;
QFile file(fileName);
if (!file.open(QIODevice::ReadOnly))
{
qDebug() << "Failed to open file: " << fileName;
return QDomElement();
}
if (!xml.setContent(&file))
{
qDebug() << "Failed to parse XML file: " << fileName;
file.close();
return QDomElement();
}
file.close();
return xml.documentElement();
}
// 保存xml文件
bool saveXmlFile(QDomElement& root, const QString& fileName)
{
QFile file(fileName);
if (!file.open(QIODevice::WriteOnly))
{
qDebug() << "Failed to open file: " << fileName;
return false;
}
QTextStream out(&file);
root.ownerDocument().save(out, 4);
file.close();
return true;
}
// 新增节点
QDomElement addNode(QDomElement& parent, const QString& tagName)
{
QDomDocument doc = parent.ownerDocument();
QDomElement newElement = doc.createElement(tagName);
parent.appendChild(newElement);
return newElement;
}
// 删除节点
void deleteNode(QDomElement& node)
{
while (!node.isNull() && node.hasChildNodes())
{
QDomNode child = node.firstChild();
node.removeChild(child);
}
QDomNode parent = node.parentNode();
parent.removeChild(node);
}
// 编辑节点
void editNode(QDomElement& node, const QString& attrName, const QString& newValue)
{
node.setAttribute(attrName, newValue);
}
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
QDomElement root = readXmlFile("test.xml");
// 新增节点
QDomElement variant = addNode(root, "variant");
variant.setAttribute("id", "1");
variant.setAttribute("name", "Variant 1");
// 删除节点
QDomElement node = root.firstChildElement("category");
deleteNode(node);
// 编辑节点
QDomElement product = root.firstChildElement("product");
editNode(product, "name", "New Product Name");
// 保存xml文件
if (!saveXmlFile(root, "test.xml"))
{
qDebug() << "Failed to save XML file.";
return -1;
}
return a.exec();
}
```
这段代码首先实现了读取xml文件并返回根节点的函数`readXmlFile`和保存xml文件的函数`saveXmlFile`。然后,实现了新增节点的函数`addNode`,该函数接受一个父节点和一个tagName参数,返回新增的节点;实现了删除节点的函数`deleteNode`,该函数接受一个节点参数,并删除该节点及其所有子节点;实现了编辑节点的函数`editNode`,该函数接受一个节点、一个属性名和一个新的属性值,用于修改节点属性的value值。
最后在`main`函数中,读取xml文件,新增、删除和编辑节点,并保存修改后的xml文件。
阅读全文
相关推荐
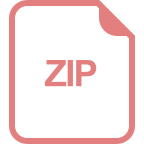
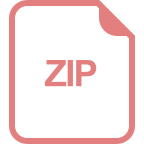















