java结合opcua实现节点以及子节点读取
时间: 2023-07-18 11:33:06 浏览: 63
您好,如果您要在Java中使用OPC UA实现节点以及子节点的读取,可以使用Eclipse Milo这个Java实现的OPC UA库。以下是一个读取节点及其子节点的示例代码:
```java
import org.eclipse.milo.opcua.sdk.client.OpcUaClient;
import org.eclipse.milo.opcua.sdk.client.api.UaBrowseResult;
import org.eclipse.milo.opcua.sdk.client.api.nodes.Node;
import org.eclipse.milo.opcua.sdk.client.nodes.UaNode;
import org.eclipse.milo.opcua.stack.core.Identifiers;
import org.eclipse.milo.opcua.stack.core.types.builtin.NodeId;
import org.eclipse.milo.opcua.stack.core.types.builtin.QualifiedName;
import org.eclipse.milo.opcua.stack.core.types.builtin.Variant;
import org.eclipse.milo.opcua.stack.core.types.enumerated.BrowseDirection;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
public class ReadNodesExample {
public static void main(String[] args) throws Exception {
// 创建OPC UA客户端
OpcUaClient client = createClient();
// 连接到OPC UA服务器
client.connect().get();
try {
// 获取根节点
NodeId rootNodeId = Identifiers.RootFolder;
UaNode rootNode = client.getAddressSpace().getNode(rootNodeId).get();
// 读取根节点的DisplayName属性
String rootDisplayName = rootNode.getDisplayName().getText();
System.out.println("Root Node DisplayName: " + rootDisplayName);
// 浏览根节点的子节点,并输出子节点的DisplayName属性
List<Node> childNodes = browseNode(client, rootNodeId);
for (Node childNode : childNodes) {
String childDisplayName = childNode.getDisplayName().getText();
System.out.println("Child Node DisplayName: " + childDisplayName);
}
} finally {
// 断开连接
client.disconnect().get();
}
}
private static OpcUaClient createClient() throws Exception {
// TODO: 替换成实际的OPC UA服务器地址
String serverUrl = "opc.tcp://localhost:4840";
// 创建OPC UA客户端
OpcUaClient client = new OpcUaClient(serverUrl);
return client;
}
private static List<Node> browseNode(OpcUaClient client, NodeId nodeId) throws ExecutionException, InterruptedException {
// 浏览节点的子节点
UaBrowseResult browseResult = client.browse(nodeId, BrowseDirection.Forward, Identifiers.References, true).get();
// 获取子节点的NodeIds
List<NodeId> childNodeIds = browseResult.getReferences().stream()
.map(reference -> reference.getReferenceTypeId().equals(Identifiers.HasComponent) ? reference.getTargetNodeId() : null)
.filter(nodeId -> nodeId != null)
.collect(Collectors.toList());
// 获取子节点的详细信息
CompletableFuture<List<Node>> future = client.getAddressSpace().getNodes(childNodeIds);
return future.get();
}
}
```
在这个示例代码中,我们首先创建了一个OPC UA客户端,连接到了OPC UA服务器。然后,我们获取了根节点并读取了根节点的DisplayName属性。接下来,我们使用browseNode()方法浏览了根节点的子节点,并输出了子节点的DisplayName属性。
需要注意的是,在实际应用中,我们应该将OPC UA客户端的创建、连接、断开连接等操作放在try-catch-finally块中,以确保在发生异常时能够正确地关闭连接。同时,我们也应该根据实际需求修改代码中的节点Id、属性名称等参数。
相关推荐
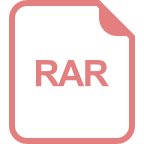













