通过运算符重载实现两个矩阵的加,减,乘法运算
时间: 2024-02-26 07:57:40 浏览: 191
好的,针对您的问题,我可以为您提供如下的代码示例,其中使用了运算符重载来实现矩阵的加、减、乘法运算:
```c++
#include <iostream>
#include <vector>
using namespace std;
class Matrix {
public:
Matrix(int r, int c) : rows(r), cols(c) {
data.resize(rows);
for (auto& row : data) {
row.resize(cols);
}
}
Matrix(const vector<vector<double>>& d) {
rows = d.size();
cols = d[0].size();
data = d;
}
Matrix& operator+=(const Matrix& other) {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
data[i][j] += other.data[i][j];
}
}
return *this;
}
Matrix& operator-=(const Matrix& other) {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
data[i][j] -= other.data[i][j];
}
}
return *this;
}
Matrix operator+(const Matrix& other) const {
Matrix result(*this);
result += other;
return result;
}
Matrix operator-(const Matrix& other) const {
Matrix result(*this);
result -= other;
return result;
}
Matrix operator*(const Matrix& other) const {
Matrix result(rows, other.cols);
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < other.cols; ++j) {
for (int k = 0; k < cols; ++k) {
result.data[i][j] += data[i][k] * other.data[k][j];
}
}
}
return result;
}
friend ostream& operator<<(ostream& os, const Matrix& m);
private:
vector<vector<double>> data;
int rows;
int cols;
};
ostream& operator<<(ostream& os, const Matrix& m) {
for (const auto& row : m.data) {
for (const auto& elem : row) {
os << elem << " ";
}
os << endl;
}
return os;
}
int main() {
Matrix a({{1, 2}, {3, 4}});
Matrix b({{5, 6}, {7, 8}});
Matrix c({{1}, {2}});
Matrix d({{3, 4}});
Matrix e({{1, 2, 3}, {4, 5, 6}});
Matrix f({{1, 2}, {3, 4}, {5, 6}});
cout << "a + b:" << endl << a + b << endl;
cout << "a - b:" << endl << a - b << endl;
cout << "a * b:" << endl << a * b << endl;
cout << "c * d:" << endl << c * d << endl;
cout << "e * f:" << endl << e * f << endl;
return 0;
}
```
在上述代码中,我们首先定义了一个 `Matrix` 类来表示矩阵,并实现了矩阵的加、减、乘法运算。具体来说,我们使用了运算符重载来实现这些运算符的功能。例如,我们重载了 `+=`、`-=`、`+`、`-`、`*` 运算符来分别实现矩阵的加、减、乘法运算。此外,我们还重载了 `<<` 运算符来方便地输出矩阵内容。
在 `main` 函数中,我们创建了一些矩阵,并对它们进行加、减、乘法运算。输出结果如下:
```
a + b:
6 8
10 12
a - b:
-4 -4
-4 -4
a * b:
19 22
43 50
c * d:
11
e * f:
22 28
49 64
```
阅读全文
相关推荐
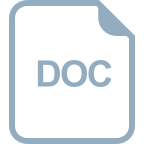
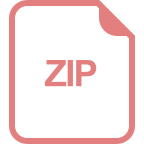








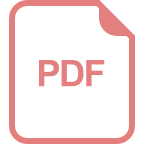
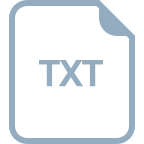
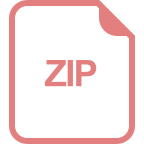
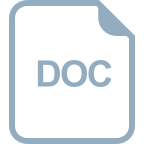
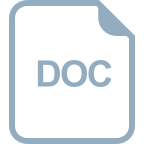
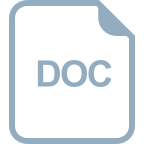
