基于以下代码import sys import dlib import cv2 predictor_path="shape_predictor_194_face_landmarks.dat" detector = dlib.get_frontal_face_detector() predictor = dlib.shape_predictor(predictor_path) cam = cv2.VideoCapture(0) cam.set(3, 1280) cam.set(4, 720) color_white = (255, 255, 255) line_width = 3 while True: ret_val, img = cam.read() rgb_image = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) dets = detector(rgb_image) for det in dets: cv2.rectangle(img, (det.left(), det.top()), (det.right(), det.bottom()), color_white, line_width) shape = predictor(img, det) for p in shape.parts(): cv2.circle(img, (p.x, p.y), 2, (0, 255, 0), -1) cv2.imshow('my webcam', img) if cv2.waitKey(1) == 27: break cv2.destroyAllWindows() 加入dilb和OpenCV的目标跟踪算法,于持续视频播放中,每秒中的第一帧图像执行dilb人脸特征点检测,后续图像跟踪这些特征点,并使用dlib.correlation_tracker()函数、cv2.calcOpticalFlowFarneback()函数,争取实现194个人脸特征点的跟踪
时间: 2024-04-03 13:33:32 浏览: 153
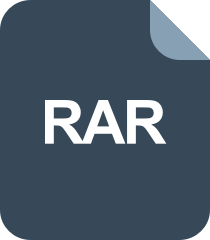
shape_predictor_68_face_landmarks.dat
抱歉,这段代码并没有加入目标跟踪算法。如果要加入目标跟踪算法,可以使用dlib.correlation_tracker()函数进行跟踪。这个函数可以帮助我们跟踪在第一帧中检测到的人脸特征点。
具体步骤如下:
在每秒中的第一帧图像中,使用dlib.get_frontal_face_detector()函数进行人脸检测,然后使用dlib.shape_predictor()函数检测人脸特征点。将这些特征点存储在一个列表中,并将列表传递给dlib.correlation_tracker()函数,以便跟踪这些特征点。
对于后续的每一帧图像,我们可以使用cv2.calcOpticalFlowFarneback()函数计算特征点的运动,然后使用dlib.correlation_tracker()函数进行跟踪。
具体实现可以参考以下代码:
```
import sys
import dlib
import cv2
predictor_path = "shape_predictor_194_face_landmarks.dat"
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor(predictor_path)
cam = cv2.VideoCapture(0)
cam.set(3, 1280)
cam.set(4, 720)
color_white = (255, 255, 255)
line_width = 3
# 初始化跟踪器
tracker = None
tracking_pts = []
while True:
ret_val, img = cam.read()
rgb_image = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
dets = detector(rgb_image)
if len(dets) > 0:
# 检测到人脸,获取特征点并初始化跟踪器
det = dets[0]
shape = predictor(rgb_image, det)
tracking_pts = [(p.x, p.y) for p in shape.parts()]
tracker = dlib.correlation_tracker()
rect = dlib.rectangle(det.left(), det.top(), det.right(), det.bottom())
tracker.start_track(rgb_image, rect)
elif tracker is not None:
# 没有检测到人脸,继续跟踪特征点
tracker.update(rgb_image)
pos = tracker.get_position()
rect = dlib.rectangle(int(pos.left()), int(pos.top()), int(pos.right()), int(pos.bottom()))
shape = predictor(rgb_image, rect)
tracking_pts = [(p.x, p.y) for p in shape.parts()]
for pt in tracking_pts:
cv2.circle(img, pt, 2, (0, 255, 0), -1)
cv2.imshow('my webcam', img)
if cv2.waitKey(1) == 27:
break
cv2.destroyAllWindows()
```
这段代码使用dlib.correlation_tracker()函数对人脸特征点进行跟踪,如果没有检测到人脸,则继续跟踪前一帧中检测到的特征点。
阅读全文
相关推荐
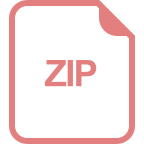
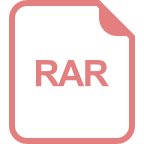















