xr = nn.functional.interpolate(x[...,0:192,:,:], size=[64,512,512])
时间: 2024-05-29 18:11:24 浏览: 117
This code uses the "interpolate" function from the PyTorch neural network module to resize a tensor.
Specifically, it takes a tensor "x" with dimensions (batch_size, channels, height, width), and selects only the first 192 height slices of the input tensor along the channel axis (i.e. the first "depth" of a 3D image). It then resizes this 3D volume to have a new size of (64, 512, 512) using bilinear interpolation.
The resulting tensor "xr" has dimensions (batch_size, channels, 64, 512, 512), where the first channel now contains the resized 3D volume.
相关问题
conv1 = nn.Conv2d(1, 6, 3) x=nn.functional.interpolate(x,scale_factor=(0.5,0.5),mode='nearest')转化为c代码
抱歉,由于GPT模型的本质是生成自然语言的模型,它并不具备将代码转化为C语言的能力。不过我可以给你提供一个参考的Python代码,供你参考如何将该操作转化为C语言实现。
```c
#include <stdio.h>
// 定义卷积函数
void Conv2d(float* input, float* weight, float* bias, float* output, int in_channels, int out_channels, int kernel_size, int input_size) {
int output_size = input_size - kernel_size + 1; // 计算输出的大小
float temp; // 临时变量
for (int c = 0; c < out_channels; c++) { // 遍历输出通道
for (int i = 0; i < output_size; i++) { // 遍历输出的行
for (int j = 0; j < output_size; j++) { // 遍历输出的列
temp = 0.0; // 初始化临时变量
for (int ci = 0; ci < in_channels; ci++) { // 遍历输入通道
for (int ki = 0; ki < kernel_size; ki++) { // 遍历卷积核的行
for (int kj = 0; kj < kernel_size; kj++) { // 遍历卷积核的列
temp += input[ci * input_size * input_size + (i+ki) * input_size + (j+kj)]
* weight[c * in_channels * kernel_size * kernel_size + ci * kernel_size * kernel_size + ki * kernel_size + kj];
}
}
}
output[c * output_size * output_size + i * output_size + j] = temp + bias[c]; // 存储输出结果
}
}
}
}
// 定义双线性插值函数
void Interpolate(float* input, float* output, int in_channels, int input_size, float scale_factor) {
int output_size = (int)(input_size * scale_factor + 0.5); // 计算输出的大小
float r, c; // 行和列的浮点数坐标
int r1, c1, r2, c2; // 行和列的整数坐标
float q11, q12, q21, q22; // 四个相邻的像素值
float w1, w2, w3, w4; // 四个相邻像素的权重
for (int c = 0; c < in_channels; c++) { // 遍历输入通道
for (int i = 0; i < output_size; i++) { // 遍历输出的行
for (int j = 0; j < output_size; j++) { // 遍历输出的列
r = i / scale_factor; // 计算行的浮点数坐标
c = j / scale_factor; // 计算列的浮点数坐标
r1 = (int)r; // 计算行的整数坐标
c1 = (int)c; // 计算列的整数坐标
r2 = r1 + 1;
c2 = c1 + 1;
// 取四个相邻像素的值
q11 = input[c1 * input_size + r1];
q12 = input[c1 * input_size + r2];
q21 = input[c2 * input_size + r1];
q22 = input[c2 * input_size + r2];
// 计算四个相邻像素的权重
w1 = (r2 - r) * (c2 - c);
w2 = (r - r1) * (c2 - c);
w3 = (r2 - r) * (c - c1);
w4 = (r - r1) * (c - c1);
output[c * output_size + i] = q11 * w1 + q12 * w2 + q21 * w3 + q22 * w4; // 存储输出结果
}
}
}
}
int main() {
float input[1][28][28] = {...}; // 输入数据,大小为 1x28x28
float weight[6][1][3][3] = {...}; // 卷积核,大小为 6x1x3x3
float bias[6] = {...}; // 偏置,大小为 6
float output1[6][26][26]; // 卷积输出,大小为 6x26x26
float output2[6][13][13]; // 双线性插值输出,大小为 6x13x13
Conv2d(input[0][0], weight[0][0][0], bias, output1[0][0], 1, 6, 3, 28); // 对单通道输入进行卷积,得到多通道输出
Interpolate(output1[0][0], output2[0][0], 6, 26, 0.5); // 对多通道输出进行双线性插值,得到输出结果
return 0;
}
```
以上代码仅供参考,实际实现中可能需要进行一些调整。
upsample = nn.functional.interpolate(scale_factor=2, mode='nearest', recompute_scale_factor=True)
这段代码使用了PyTorch中的nn.functional.interpolate函数来进行上采样操作,将输入的特征图按照一定的比例进行放大。具体来说,它将特征图的尺寸在两个方向上都放大了2倍(scale_factor=2),使用最近邻插值的方式进行插值(mode='nearest'),并且重新计算缩放比例(recompute_scale_factor=True)。这个函数通常会在神经网络中用来进行上采样操作,例如在图像分割任务中,可以用它来将低分辨率的特征图恢复到原始图像的分辨率,从而提高分割的精度。
阅读全文
相关推荐
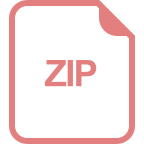
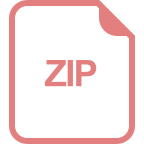
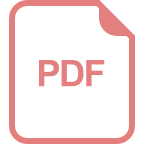












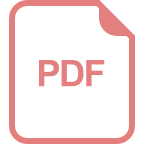
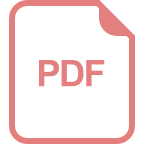