def forward(self, x, flow, scale): if scale != 1: x = F.interpolate(x, scale_factor = 1. / scale, mode="bilinear", align_corners=False) if flow != None: flow = F.interpolate(flow, scale_factor = 1. / scale, mode="bilinear", align_corners=False) * 1. / scale x = torch.cat((x, flow), 1) x = self.conv0(x) x = self.convblock(x) + x tmp = self.lastconv(x) tmp = F.interpolate(tmp, scale_factor = scale * 2, mode="bilinear", align_corners=False) flow = tmp[:, :4] * scale * 2 mask = tmp[:, 4:5] return flow, mask翻译代码
时间: 2023-12-29 08:04:00 浏览: 172
这段代码是一个神经网络模型的前向传播函数。它接受三个输入参数:x,flow和scale。根据scale的值是否等于1,对输入x进行插值操作,将其缩放到1/scale的尺寸。如果flow不为None,则对其进行同样的插值操作,并将其缩放到1/scale的尺寸后与x进行拼接。接下来,将拼接后的结果传入conv0进行卷积操作,然后经过convblock进行卷积操作,并将其与x相加。然后,将结果传入lastconv进行卷积操作得到tmp。对tmp再次进行插值操作,将其缩放到scale乘以2的尺寸。最后,取出tmp中的前四列数据,乘以scale乘以2得到flow,并取出tmp中的第5列数据得到mask。函数返回flow和mask作为输出结果。
相关问题
解释一下这段代码def forward(self, x): assert x.shape[1] == self.channels if self.dims == 3: x = F.interpolate( x, (x.shape[2], x.shape[3] * 2, x.shape[4] * 2), mode="nearest" ) else: x = F.interpolate(x, scale_factor=2, mode="nearest") if self.use_conv: x = self.conv(x) return x
这段代码是一个神经网络模型中的前向传播函数,输入参数 x 是一个张量,函数首先会检查 x 的第二个维度是否等于模型中指定的通道数,如果不等于则会报错。接着,如果模型是三维的,则会对 x 进行插值操作,将其在第三个和第四个维度上分别扩大两倍,保持第二个维度不变;如果模型是二维的,则会将 x 在两个维度上分别扩大两倍。最后,如果模型中指定了使用卷积层,则会对 x 进行一次卷积操作,最终返回处理后的张量 x。
分析这个代码class OhemCrossEntropy(nn.Module): def __init__(self, ignore_label=-1, thres=0.7, min_kept=100000, weight=None): super(OhemCrossEntropy, self).__init__() self.thresh = thres self.min_kept = max(1, min_kept) self.ignore_label = ignore_label self.criterion = nn.CrossEntropyLoss( weight=weight, ignore_index=ignore_label, reduction='none' ) def _ce_forward(self, score, target): ph, pw = score.size(2), score.size(3) h, w = target.size(1), target.size(2) if ph != h or pw != w: score = F.interpolate(input=score, size=( h, w), mode='bilinear', align_corners=config.MODEL.ALIGN_CORNERS) loss = self.criterion(score, target) return loss def _ohem_forward(self, score, target, **kwargs): ph, pw = score.size(2), score.size(3) h, w = target.size(1), target.size(2) if ph != h or pw != w: score = F.interpolate(input=score, size=( h, w), mode='bilinear', align_corners=config.MODEL.ALIGN_CORNERS) pred = F.softmax(score, dim=1) pixel_losses = self.criterion(score, target).contiguous().view(-1) mask = target.contiguous().view(-1) != self.ignore_label tmp_target = target.clone() tmp_target[tmp_target == self.ignore_label] = 0 pred = pred.gather(1, tmp_target.unsqueeze(1)) pred, ind = pred.contiguous().view(-1,)[mask].contiguous().sort() min_value = pred[min(self.min_kept, pred.numel() - 1)] threshold = max(min_value, self.thresh) pixel_losses = pixel_losses[mask][ind] pixel_losses = pixel_losses[pred < threshold] return pixel_losses.mean() def forward(self, score, target): if config.MODEL.NUM_OUTPUTS == 1: score = [score] weights = config.LOSS.BALANCE_WEIGHTS assert len(weights) == len(score) functions = [self._ce_forward] * \ (len(weights) - 1) + [self._ohem_forward] return sum([ w * func(x, target) for (w, x, func) in zip(weights, score, functions) ])
这是一个实现了OHEM(Online Hard Example Mining)的交叉熵损失函数,用于解决深度学习中存在难样本或噪声样本导致训练效果不好的问题。其中,thresh表示像素的softmax预测概率阈值,小于该阈值的像素被认为是难样本;min_kept表示每张图中保留的难样本最小数量;ignore_label表示忽略的标签;weight表示权重。_ce_forward函数实现了普通的交叉熵损失的计算;_ohem_forward函数实现了OHEM的计算。在forward函数中,对于有多个输出的模型,采用了权重平衡的方式进行计算。
阅读全文
相关推荐
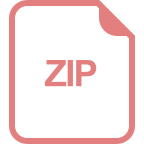
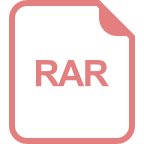
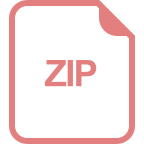














