def forward_features(self, x): x, B, T = self.patch_embed(x) # x in shape (BT, HW, C) if self.ape: x = x + self.absolute_pos_embed ## Add temporal embedding if not self.t_relative: x = rearrange(x, '(b t) n c -> (b n) t c', b=B, t=T) x = x + self.temporal_embedding x = rearrange(x, '(b n) t c -> (b t) n c', b=B, t=T) x = self.pos_drop(x) for layer in self.layers: x = layer(x) x = self.norm(x) ## Final temporal block H = self.layers[-1].input_resolution[0] x = rearrange(x, '(B T) (H W) C -> B C T H W', T=T, H=H) # x = torch.mean(x, (3, 4)) # x = rearrange(x, 'B C T -> B T C') # x = self.blocks_t(x) # x = self.norm(x) # x = rearrange(x, 'B T C -> B C T') # x = x.unsqueeze(-1).unsqueeze(-1) # x = self.avgpool(x.transpose(1, 2)) # B C 1 # x = torch.flatten(x, 1) return x
时间: 2023-06-19 09:06:25 浏览: 268
这是一个PyTorch模型中的forward方法的一部分。它接受输入x,并将其传递到模型的不同层和块中进行处理。在这个方法中,输入x首先通过patch_embed模块进行处理,然后进行绝对位置编码和时间编码的处理。然后,输入通过一系列的层和块进行处理,最终通过一些后续的操作(例如平均池化和展平)产生输出。这个方法的输出形状为BCTHW,其中B是批次大小,T是时间步数,H和W是空间维度,C是通道数。
相关问题
import torch.nn as nnclass ViT(nn.Module): def __init__(self, img_size, patch_size, num_classes, dim): super().__init__() self.patch_size = patch_size num_patches = (img_size // patch_size) ** 2 patch_dim = 3 * patch_size ** 2 # 输入的通道数,3表示RGB通道 self.class_embed = nn.Parameter(torch.randn(1, 1, dim)) self.patch_embed = nn.Linear(patch_dim, dim) self.pos_embed = nn.Parameter(torch.randn(1, num_patches + 1, dim)) self.cls_token = nn.Parameter(torch.randn(1, 1, dim)) self.transformer = nn.TransformerEncoderLayer(d_model=dim, nhead=8) self.linear = nn.Linear(dim, num_classes) def forward(self, x): batch_size, _, _, _ = x.shape patches = x.unfold(2, self.patch_size, self.patch_size).unfold(3, self.patch_size, self.patch_size) patches = patches.flatten(2).transpose(1, 2) patch_embed = self.patch_embed(patches) pos_embed = self.pos_embed[:, :(patches.size(1) + 1)] cls_tokens = self.cls_token.expand(batch_size, -1, -1) x = torch.cat([cls_tokens, patch_embed], dim=1) x += pos_embed x = self.transformer(x) x = x.mean(dim=1) x = self.linear(x) return x
这段代码是用来定义一个名为ViT的类,其继承自nn.Module。其有四个参数,包括图像尺寸img_size、每个patch的尺寸patch_size、类别数目num_classes和维度dim。在初始化时,代码调用了父类的构造函数,并且将patch_size保存在self.patch_size中。由于图像被切成了多个patch,因此需要计算patch的数目num_patches,以及每个patch的维度patch_dim。
def flops(self): flops = 0 flops += self.patch_embed.flops() for i, layer in enumerate(self.layers): flops += layer.flops() # flops += self.num_features * self.patches_resolution[0] * self.patches_resolution[1] // (2 ** self.num_layers) # flops += self.num_features * self.num_classes return flops
这是一个类中的方法,用于计算模型的 FLOPs(floating-point operations,即浮点运算量),可以用于评估模型的计算复杂度。具体实现中,首先计算输入图像的 Patch Embedding 的 FLOPs,然后循环遍历所有的 Transformer 层,计算每一层的 FLOPs 并累加到总的 FLOPs 中。最后,还可以选择性地加上最后一层全连接层的计算 FLOPs。
阅读全文
相关推荐
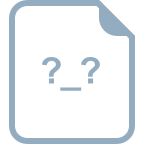
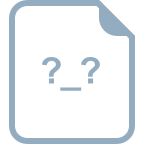
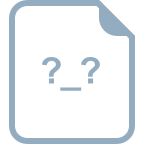













