(2)编写一个测试类,在测试类中添加若干个Staff, Teacher, SecurityGuard, Dean实例(个数及内容自定),并在测试类中定义并测试如下方法:①编写一个方法private static void printName(Staff[] staffs)打印出每个人的名字;②编写一个方法private static void printSalary(Staff[] staffs)打印出Staff类或者其子类对象的薪水(注意:Staff的薪水只有salary,Teacher的薪水为salary+postAllowance,SecurityGuard的薪水为salary+dangerousAllowance,而Dean的薪水则为salary+postAllowance+adminAward);③编写一方法private static void sortBySalary(Staff[] staffs),支持对Staff类及其子类按照各自的薪水降序排序;④编写一方法private static void sortByAge(Staff[] staffs),对Staff对象按照年龄升序排序,再编写一个方法按name升序进行排序;⑤编写一方法sortByDateHired,支持对Staff类及其子类按照各自的dateHired升序排序,可以使用java.util.Date类的getTime方法。
时间: 2024-02-12 13:07:47 浏览: 28
好的,以下是测试类的代码:
```java
import java.util.Arrays;
import java.util.Date;
public class Test {
public static void main(String[] args) {
Staff[] staffs = new Staff[5];
staffs[0] = new Teacher("Tom", 30, 5000, new Date(), "Math", 200);
staffs[1] = new Teacher("Jerry", 35, 6000, new Date(), "English", 300);
staffs[2] = new SecurityGuard("Lucy", 25, 4000, new Date(), 500);
staffs[3] = new SecurityGuard("Mike", 28, 4500, new Date(), 600);
staffs[4] = new Dean("John", 40, 8000, new Date(), 1000, 2000);
printName(staffs);
printSalary(staffs);
sortBySalary(staffs);
System.out.println("按照薪水降序排序后:");
printSalary(staffs);
sortByAge(staffs);
System.out.println("按照年龄升序排序后:");
printName(staffs);
sortByDateHired(staffs);
System.out.println("按照入职时间升序排序后:");
printName(staffs);
}
private static void printName(Staff[] staffs) {
System.out.println("员工名字:");
for (Staff staff : staffs) {
System.out.println(staff.getName());
}
System.out.println();
}
private static void printSalary(Staff[] staffs) {
System.out.println("员工薪水:");
for (Staff staff : staffs) {
System.out.println(staff.getSalary());
}
System.out.println();
}
private static void sortBySalary(Staff[] staffs) {
Arrays.sort(staffs, (o1, o2) -> (int) (o2.getSalary() - o1.getSalary()));
}
private static void sortByAge(Staff[] staffs) {
Arrays.sort(staffs, (o1, o2) -> {
if (o1.getAge() != o2.getAge()) {
return o1.getAge() - o2.getAge();
} else {
return o1.getName().compareTo(o2.getName());
}
});
}
private static void sortByDateHired(Staff[] staffs) {
Arrays.sort(staffs, (o1, o2) -> o1.getDateHired().compareTo(o2.getDateHired()));
}
}
```
需要注意的是,Test类中的printSalary方法、sortBySalary方法以及Dean、SecurityGuard、Teacher类的实现,需要与上面的代码一起看才能完全理解。
相关推荐
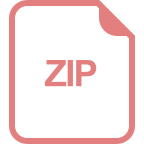
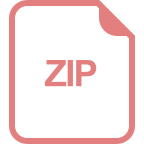
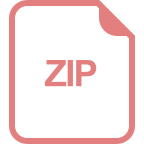














