C语言长整数处理运算代码
时间: 2023-11-12 18:10:24 浏览: 40
下面是C语言实现的长整数加法和乘法运算代码:
```c
#include<stdio.h>
#include<string.h>
// 定义最大位数,一般为10的整数次幂
#define MAX_LEN 1000
// 长整数结构体
struct bigInt {
int digit[MAX_LEN]; // 存储每一位的值
int size; // 当前位数
};
// 初始化长整数
void init(struct bigInt *a) {
memset(a->digit, 0, sizeof(a->digit));
a->size = 0;
}
// 将字符串转换为长整数
void strToInt(struct bigInt *a, char str[]) {
init(a);
int len = strlen(str);
for(int i = len - 1, j = 0, t = 0, c = 1; i >= 0; i--) {
t += (str[i] - '0') * c;
j++;
c *= 10;
if(j == 4 || i == 0) { // 每四位处理一次
a->digit[a->size++] = t;
j = 0;
t = 0;
c = 1;
}
}
}
// 比较两个长整数的大小,a > b 返回 1,a == b 返回 0,a < b 返回 -1
int compare(struct bigInt a, struct bigInt b) {
if(a.size > b.size) return 1;
if(a.size < b.size) return -1;
for(int i = a.size - 1; i >= 0; i--) {
if(a.digit[i] > b.digit[i]) return 1;
if(a.digit[i] < b.digit[i]) return -1;
}
return 0;
}
// 长整数加法
struct bigInt add(struct bigInt a, struct bigInt b) {
struct bigInt c;
init(&c);
int carry = 0; // 进位
for(int i = 0; i < a.size || i < b.size; i++) {
int sum = a.digit[i] + b.digit[i] + carry;
c.digit[c.size++] = sum % 10000; // 每四位分开存储
carry = sum / 10000;
}
if(carry != 0) { // 处理最高位进位
c.digit[c.size++] = carry;
}
return c;
}
// 长整数乘法
struct bigInt mul(struct bigInt a, struct bigInt b) {
struct bigInt c;
init(&c);
for(int i = 0; i < a.size; i++) {
int carry = 0;
for(int j = 0; j < b.size; j++) {
int sum = a.digit[i] * b.digit[j] + carry + c.digit[i+j];
c.digit[i+j] = sum % 10000;
carry = sum / 10000;
}
if(carry != 0) {
c.digit[i + b.size] = carry;
}
}
c.size = a.size + b.size - 1;
if(c.digit[c.size] != 0) { // 处理最高位进位
c.size++;
}
return c;
}
// 输出长整数
void print(struct bigInt a) {
printf("%d", a.digit[a.size - 1]);
for(int i = a.size - 2; i >= 0; i--) {
printf("%04d", a.digit[i]); // 不足四位前面补0
}
printf("\n");
}
int main() {
// 测试代码
struct bigInt a, b, c;
char str1[] = "12345678901234567890";
char str2[] = "98765432109876543210";
strToInt(&a, str1);
strToInt(&b, str2);
c = add(a, b);
printf("Addition: ");
print(c);
c = mul(a, b);
printf("Multiplication: ");
print(c);
return 0;
}
```
上述代码中,使用结构体 `bigInt` 存储长整数,其中 `digit` 数组存储每一位的值,`size` 表示当前位数。`strToInt` 函数将字符串转换为长整数,`compare` 函数比较两个长整数的大小,`add` 函数实现长整数加法,`mul` 函数实现长整数乘法,`print` 函数输出长整数。需要注意的是,因为每四位分开存储,因此在输出时需要特殊处理。
相关推荐
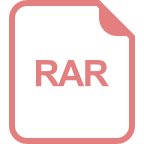














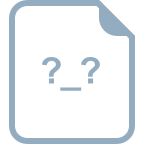