dqn路径规划pytorch
时间: 2023-09-08 14:09:37 浏览: 293
DQN(Deep Q-Network)是一种基于深度学习的增强学习算法,可以用于路径规划任务。在使用PyTorch实现DQN路径规划时,你可以按照以下步骤进行:
1. 导入必要的库:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
```
2. 定义Q网络:
```python
class QNetwork(nn.Module):
def __init__(self, state_size, action_size):
super(QNetwork, self).__init__()
self.fc1 = nn.Linear(state_size, 64)
self.fc2 = nn.Linear(64, 64)
self.fc3 = nn.Linear(64, action_size)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = torch.relu(self.fc2(x))
x = self.fc3(x)
return x
```
3. 定义DQN Agent:
```python
class DQNAgent:
def __init__(self, state_size, action_size):
self.state_size = state_size
self.action_size = action_size
self.q_network = QNetwork(state_size, action_size)
self.optimizer = optim.Adam(self.q_network.parameters(), lr=0.001)
def get_action(self, state, epsilon):
if np.random.rand() <= epsilon:
return np.random.choice(self.action_size)
else:
state = torch.from_numpy(state).float().unsqueeze(0)
q_values = self.q_network(state)
return torch.argmax(q_values).item()
def train(self, state, action, reward, next_state, done):
state = torch.from_numpy(state).float().unsqueeze(0)
next_state = torch.from_numpy(next_state).float().unsqueeze(0)
action = torch.tensor(action).unsqueeze(0)
reward = torch.tensor(reward).unsqueeze(0)
done = torch.tensor(done).unsqueeze(0)
q_values = self.q_network(state)
next_q_values = self.q_network(next_state)
target = reward + (1 - done) * torch.max(next_q_values, dim=1)[0]
target = target.detach()
loss = nn.MSELoss()(q_values.gather(1, action.unsqueeze(1)), target.unsqueeze(1))
self.optimizer.zero_grad()
loss.backward()
self.optimizer.step()
```
4. 定义训练循环:
```python
env = YourEnvironment() # 替换为你的环境类
state_size = env.state_size
action_size = env.action_size
agent = DQNAgent(state_size, action_size)
episodes = 1000
epsilon = 1.0
epsilon_decay = 0.999
epsilon_min = 0.01
batch_size = 32
for episode in range(episodes):
state = env.reset()
total_reward = 0
while True:
action = agent.get_action(state, epsilon)
next_state, reward, done = env.step(action)
agent.train(state, action, reward, next_state, done)
state = next_state
total_reward += reward
if done:
break
epsilon *= epsilon_decay
epsilon = max(epsilon, epsilon_min)
print(f"Episode: {episode+1}, Reward: {total_reward}")
```
这是一个简单的使用PyTorch实现DQN路径规划的示例,具体的代码需要根据你的环境和任务需求进行调整。希望对你有所帮助!
阅读全文
相关推荐
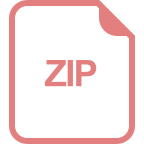
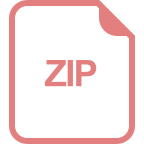

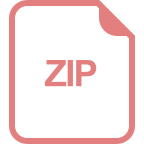
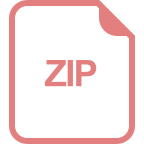
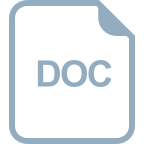





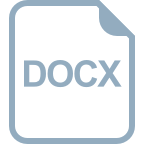
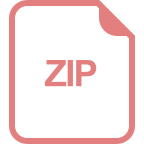
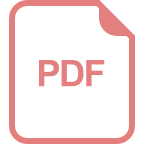
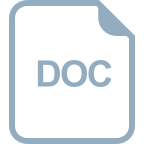
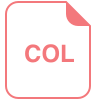

