最长公共子序列代码 C++
时间: 2024-04-14 22:23:43 浏览: 11
最长公共子序列(Longest Common Subsequence,简称LCS)是一种常见的字符串匹配问题,可以使用动态规划算法来解决。以下是一个用C++实现的LCS代码示例:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int longestCommonSubsequence(string text1, string text2) {
int m = text1.length();
int n = text2.length();
vector<vector<int>> dp(m + 1, vector<int>(n + 1, 0));
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
if (text1[i - 1] == text2[j - 1]) {
dp[i][j] = dp[i - 1][j - 1] + 1;
} else {
dp[i][j] = max(dp[i - 1][j], dp[i][j - 1]);
}
}
}
return dp[m][n];
}
int main() {
string text1 = "abcde";
string text2 = "ace";
int result = longestCommonSubsequence(text1, text2);
cout << "最长公共子序列的长度为:" << result << endl;
return 0;
}
```
这段代码中,我们定义了一个二维数组`dp`来保存最长公共子序列的长度。通过两层循环遍历字符串`text1`和`text2`,如果当前字符相等,则最长公共子序列的长度加一;否则,取前一个状态的最长公共子序列长度。最后返回`dp[m][n]`即为最长公共子序列的长度。
相关推荐
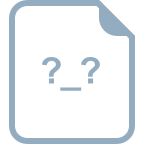
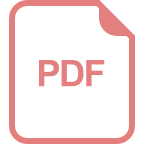














