json转为yolo格式
时间: 2023-11-17 20:04:23 浏览: 192
Yolo格式是一种用于目标检测的格式,可以将图像中的目标位置、类别等信息保存在一个文本文件中。下面是将json转为yolo格式的步骤:
1. 将json文件读入内存,解析出图像的宽度和高度,以及目标的位置、类别等信息。
2. 按照yolo格式的要求,将目标的位置、宽度和高度转换为相对于图像宽度和高度的比例。
3. 将目标类别转换为数字编码,例如将“dog”编码为“0”、“cat”编码为“1”。
4. 将转换后的数据按照yolo格式的要求,写入一个文本文件中,每个目标占据一行,格式为:
```
<object-class> <x> <y> <width> <height>
```
其中:
- <object-class>是目标的数字编码。
- <x>和<y>是目标中心点相对于图像宽度和高度的比例。
- <width>和<height>是目标的宽度和高度相对于图像宽度和高度的比例。
5. 将生成的文本文件命名与原图像相同,但后缀名为“.txt”,并保存到与原图像相同的目录下。
注意:在转换过程中需要注意保持精度,避免数据丢失或错误。同时,需要根据具体的yolo版本和配置文件,确定目标类别的编码方式和坐标格式。
相关问题
json转为yolo txt python代码
下面是将JSON转换为YOLO txt的Python代码:
```python
import json
import os
# json文件路径
json_file = 'path/to/your/json/file.json'
# 类别名
classes = ["class1", "class2", "class3"]
# yolo txt文件路径
txt_file = 'path/to/your/yolo/txt/file.txt'
# 图像路径
img_dir = 'path/to/your/image/directory'
# 读取json文件
with open(json_file, 'r') as f:
data = json.load(f)
# 解析json数据并写入yolo txt文件
with open(txt_file, 'w') as f:
for img in data:
img_filename = img['filename']
img_width = img['width']
img_height = img['height']
for obj in img['objects']:
class_name = obj['classTitle']
if class_name not in classes:
continue
class_id = classes.index(class_name)
x, y, w, h = obj['points']['exterior'][0] + obj['points']['exterior'][1]
x = x / img_width
y = y / img_height
w = w / img_width
h = h / img_height
line = str(class_id) + ' ' + str(x) + ' ' + str(y) + ' ' + str(w) + ' ' + str(h) + '\n'
f.write(os.path.join(img_dir, img_filename) + ' ' + line)
print('Convert Done!')
```
其中,需要将`path/to/your/json/file.json`、`path/to/your/yolo/txt/file.txt`和`path/to/your/image/directory`替换为实际的路径。在`classes`列表中,需要按照实际需要定义类别名。运行代码后,会在指定的路径下生成YOLO txt文件。
json转为yolo txt C++代码
以下是将JSON转换为YOLO txt的C++代码示例:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <json/json.h>
using namespace std;
// 类别名
vector<string> classes = {"class1", "class2", "class3"};
// json文件路径
string json_file = "path/to/your/json/file.json";
// yolo txt文件路径
string txt_file = "path/to/your/yolo/txt/file.txt";
// 图像路径
string img_dir = "path/to/your/image/directory";
int main() {
// 读取json文件
ifstream ifs(json_file);
Json::Value root;
ifs >> root;
// 解析json数据并写入yolo txt文件
ofstream ofs(txt_file);
for (auto img : root) {
string img_filename = img["filename"].asString();
int img_width = img["width"].asInt();
int img_height = img["height"].asInt();
for (auto obj : img["objects"]) {
string class_name = obj["classTitle"].asString();
if (find(classes.begin(), classes.end(), class_name) == classes.end()) {
continue;
}
int class_id = distance(classes.begin(), find(classes.begin(), classes.end(), class_name));
auto points = obj["points"]["exterior"];
int x1 = points[0]["x"].asInt();
int y1 = points[0]["y"].asInt();
int x2 = points[1]["x"].asInt();
int y2 = points[1]["y"].asInt();
float x = (x1 + x2) / 2.0 / img_width;
float y = (y1 + y2) / 2.0 / img_height;
float w = abs(x2 - x1) / img_width;
float h = abs(y2 - y1) / img_height;
ofs << img_dir << "/" << img_filename << " " << class_id << " " << x << " " << y << " " << w << " " << h << endl;
}
}
cout << "Convert Done!" << endl;
return 0;
}
```
注意,需要将`path/to/your/json/file.json`、`path/to/your/yolo/txt/file.txt`和`path/to/your/image/directory`替换为实际的路径。在`classes`列表中,需要按照实际需要定义类别名。代码使用了JsonCpp库来解析JSON文件,需要在项目中添加该库的头文件和链接选项。运行代码后,会在指定的路径下生成YOLO txt文件。
相关推荐
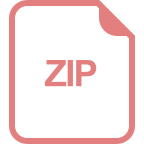







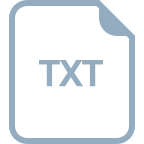
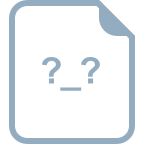
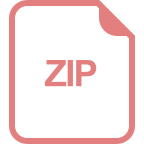
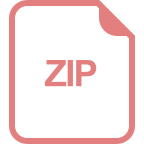