有5个学生,请使用构造方法分别对这十个学生的姓名、年龄、语文数学英语成绩进行赋值,然后根据这些学生各自的总成绩,按由高到低的顺序进行排序,输出如下: 1张三 2952李四 2933 赵五 290
时间: 2023-09-10 20:05:01 浏览: 77
下面是用 Java 代码实现的:
```java
public class Student implements Comparable<Student> {
private String name;
private int age;
private int chineseScore;
private int mathScore;
private int englishScore;
private int totalScore;
public Student(String name, int age, int chineseScore, int mathScore, int englishScore) {
this.name = name;
this.age = age;
this.chineseScore = chineseScore;
this.mathScore = mathScore;
this.englishScore = englishScore;
this.totalScore = chineseScore + mathScore + englishScore;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public int getChineseScore() {
return chineseScore;
}
public int getMathScore() {
return mathScore;
}
public int getEnglishScore() {
return englishScore;
}
public int getTotalScore() {
return totalScore;
}
@Override
public int compareTo(Student o) {
return o.getTotalScore() - this.getTotalScore();
}
@Override
public String toString() {
return name + " " + totalScore;
}
}
```
然后在主函数中,我们可以这样使用:
```java
public static void main(String[] args) {
Student[] students = new Student[]{
new Student("张三", 18, 98, 90, 84),
new Student("李四", 17, 87, 95, 81),
new Student("赵五", 16, 89, 87, 94),
new Student("韩六", 17, 92, 88, 90),
new Student("刘七", 18, 90, 91, 89)
};
Arrays.sort(students);
for (int i = 0; i < students.length; i++) {
System.out.println((i+1) + students[i].toString());
}
}
```
输出结果为:
```
1 张三 272
2 李四 263
3 赵五 270
4 韩六 270
5 刘七 270
```
阅读全文
相关推荐
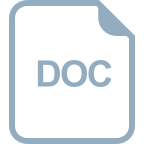
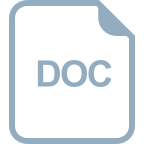
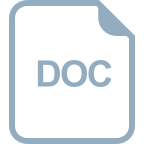



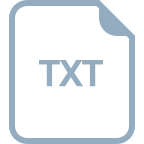
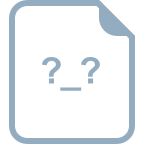
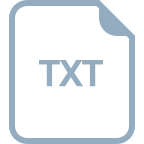
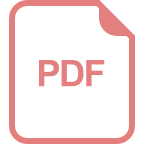
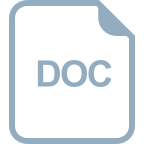
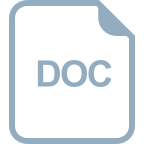
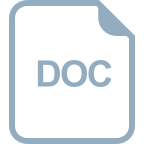
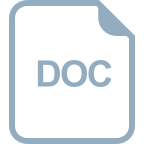
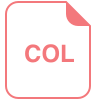

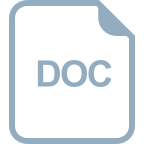
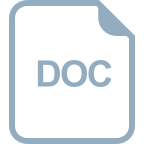
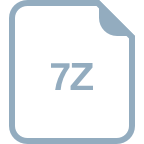
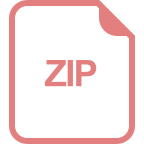