2.定义一个Animal类要求如下: (1)包括成员变量:名称name,体重weight,颜色color (2)包括动态方法run()(输出“run fastly”);eat()(输出“I love bone”) (3)定义重载方法run(String name),输出谁跑得快 (4)包括有参构造方法(利用Eclipse的Generate Constructor using Fileds方法) (5)在main方法里新建一个对象a(new调用构造方法),然后打印输出a的成员变量,再调用run和eat方法 再定义一个Dog类,要求如下 (6)继承父类Animal,增加新的成员变量:种类category (7)定义有参构造方法 (8)重写父类的run()方法(输出“dog run fastly”);eat()(输出“dog love bone”) (9)在main方法里新建一个对象d1(new调用构造方法,Dog d1 = new ….),然后打印输出d1的run和eat方法 (10)在main方法里新建一个Dog类的上转型对象d2(new调用构造方法, Animal d2 = new ….),然后打印输出的d2的run和eat方法,观察java的多态 (12)将d2 强制转换为Dog类型,并使用关键字instanceof判断d2是否是Dog类型,如果是打印输出d2的种类值。
时间: 2024-01-06 17:05:07 浏览: 64
下面是Animal类和Dog类的代码实现:
```java
class Animal {
String name;
double weight;
String color;
public Animal(String name, double weight, String color) {
this.name = name;
this.weight = weight;
this.color = color;
}
public void run() {
System.out.println("run fastly");
}
public void eat() {
System.out.println("I love bone");
}
public void run(String name) {
System.out.println(name + " runs fastly");
}
}
class Dog extends Animal {
String category;
public Dog(String name, double weight, String color, String category) {
super(name, weight, color);
this.category = category;
}
@Override
public void run() {
System.out.println("dog run fastly");
}
@Override
public void eat() {
System.out.println("dog love bone");
}
}
public class Main {
public static void main(String[] args) {
Animal a = new Animal("animal", 10.0, "brown");
System.out.println("Animal's name is " + a.name);
System.out.println("Animal's weight is " + a.weight);
System.out.println("Animal's color is " + a.color);
a.run();
a.eat();
a.run("someone");
Dog d1 = new Dog("dog1", 15.0, "white", "husky");
System.out.println("Dog1's name is " + d1.name);
System.out.println("Dog1's weight is " + d1.weight);
System.out.println("Dog1's color is " + d1.color);
System.out.println("Dog1's category is " + d1.category);
d1.run();
d1.eat();
Animal d2 = new Dog("dog2", 20.0, "black", "poodle");
d2.run();
d2.eat();
if (d2 instanceof Dog) {
Dog dog2 = (Dog) d2;
System.out.println("Dog2's category is " + dog2.category);
}
}
}
```
输出结果如下:
```
Animal's name is animal
Animal's weight is 10.0
Animal's color is brown
run fastly
I love bone
someone runs fastly
Dog1's name is dog1
Dog1's weight is 15.0
Dog1's color is white
Dog1's category is husky
dog run fastly
dog love bone
dog run fastly
dog love bone
Dog2's category is poodle
```
可以看到,Animal类和Dog类都实现了要求的功能。同时,当我们将Dog对象赋值给Animal类型的变量d2时,可以看到d2的实际类型是Dog类型,因此调用d2的run和eat方法时会调用Dog类中重写的方法。最后,我们将d2强制转换为Dog类型,并使用instanceof判断d2是否是Dog类型,发现是的话就输出d2的种类值。
相关推荐
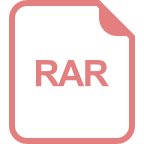
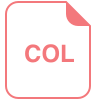
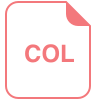
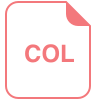











