使用c++分别采用递归和非递归算法实现树的先序,中序,后序遍历,同时实现树的层次遍历。要求:提供菜单进行选择,任给实例并演示求解过程和结果
时间: 2024-03-23 09:43:24 浏览: 49
好的,我可以帮您解答这个问题。首先,我们需要定义树的数据结构。假设我们的树是一棵二叉树,其节点结构体定义如下:
```cpp
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
```
接下来,我们可以分别实现递归和非递归算法来进行树的遍历。对于非递归算法,我们可以使用栈来模拟递归调用的过程。
**先序遍历**
递归实现:
```cpp
void PreorderTraversal(TreeNode* root) {
if (root == NULL) {
return;
}
cout << root->val << " ";
PreorderTraversal(root->left);
PreorderTraversal(root->right);
}
```
非递归实现:
```cpp
void PreorderTraversal(TreeNode* root) {
stack<TreeNode*> s;
TreeNode* p = root;
while (p != NULL || !s.empty()) {
while (p != NULL) {
cout << p->val << " ";
s.push(p);
p = p->left;
}
if (!s.empty()) {
p = s.top();
s.pop();
p = p->right;
}
}
}
```
**中序遍历**
递归实现:
```cpp
void InorderTraversal(TreeNode* root) {
if (root == NULL) {
return;
}
InorderTraversal(root->left);
cout << root->val << " ";
InorderTraversal(root->right);
}
```
非递归实现:
```cpp
void InorderTraversal(TreeNode* root) {
stack<TreeNode*> s;
TreeNode* p = root;
while (p != NULL || !s.empty()) {
while (p != NULL) {
s.push(p);
p = p->left;
}
if (!s.empty()) {
p = s.top();
s.pop();
cout << p->val << " ";
p = p->right;
}
}
}
```
**后序遍历**
递归实现:
```cpp
void PostorderTraversal(TreeNode* root) {
if (root == NULL) {
return;
}
PostorderTraversal(root->left);
PostorderTraversal(root->right);
cout << root->val << " ";
}
```
非递归实现:
```cpp
void PostorderTraversal(TreeNode* root) {
stack<TreeNode*> s;
TreeNode* p = root;
TreeNode* lastVisited = NULL;
while (p != NULL || !s.empty()) {
while (p != NULL) {
s.push(p);
p = p->left;
}
p = s.top();
if (p->right == NULL || p->right == lastVisited) {
cout << p->val << " ";
s.pop();
lastVisited = p;
p = NULL;
} else {
p = p->right;
}
}
}
```
**层次遍历**
层次遍历可以使用队列来实现。我们可以先将根节点入队,然后每次出队一个节点,访问它的值,再将其左右子节点入队。
```cpp
void LevelorderTraversal(TreeNode* root) {
queue<TreeNode*> q;
if (root != NULL) {
q.push(root);
}
while (!q.empty()) {
TreeNode* p = q.front();
q.pop();
cout << p->val << " ";
if (p->left != NULL) {
q.push(p->left);
}
if (p->right != NULL) {
q.push(p->right);
}
}
}
```
最后,我们可以编写一个菜单程序,让用户选择遍历方式和输入树的值,然后演示求解过程和结果。具体实现方式可以参考下面的代码:
```cpp
int main() {
TreeNode* root = NULL;
while (true) {
cout << "1. Preorder traversal" << endl;
cout << "2. Inorder traversal" << endl;
cout << "3. Postorder traversal" << endl;
cout << "4. Levelorder traversal" << endl;
cout << "5. Exit" << endl;
int choice;
cout << "Enter your choice: ";
cin >> choice;
if (choice == 1) {
cout << "Enter the tree values: ";
root = CreateTree();
cout << "Preorder traversal: ";
PreorderTraversal(root);
cout << endl;
} else if (choice == 2) {
cout << "Enter the tree values: ";
root = CreateTree();
cout << "Inorder traversal: ";
InorderTraversal(root);
cout << endl;
} else if (choice == 3) {
cout << "Enter the tree values: ";
root = CreateTree();
cout << "Postorder traversal: ";
PostorderTraversal(root);
cout << endl;
} else if (choice == 4) {
cout << "Enter the tree values: ";
root = CreateTree();
cout << "Levelorder traversal: ";
LevelorderTraversal(root);
cout << endl;
} else if (choice == 5) {
break;
} else {
cout << "Invalid choice!" << endl;
}
}
return 0;
}
```
其中,`CreateTree()` 函数用于创建一棵二叉树,具体实现方式可以参考下面的代码:
```cpp
TreeNode* CreateTree() {
queue<TreeNode*> q;
int val;
cin >> val;
if (val == -1) {
return NULL;
}
TreeNode* root = new TreeNode(val);
q.push(root);
while (!q.empty()) {
TreeNode* p = q.front();
q.pop();
cin >> val;
if (val != -1) {
p->left = new TreeNode(val);
q.push(p->left);
}
cin >> val;
if (val != -1) {
p->right = new TreeNode(val);
q.push(p->right);
}
}
return root;
}
```
这样,我们就完成了树的遍历算法的实现和演示。
阅读全文
相关推荐
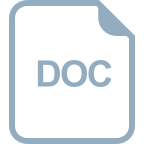
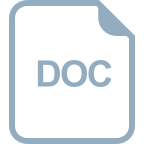
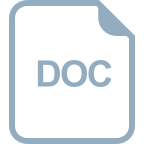
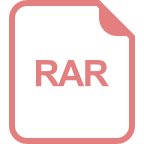

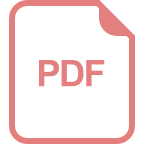
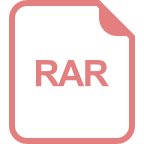
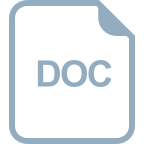








