二叉链表的递归算法:1)遍历算法(先序,中序以及后序递归遍历算法)2)统计度为1结点的个数的C++代码
时间: 2023-06-25 14:07:16 浏览: 51
1)二叉链表的递归遍历算法:
先序遍历:
```c++
void PreOrder(BiTree T) {
if(T != NULL) {
printf("%c", T->data); // 访问根节点
PreOrder(T->lchild); // 遍历左子树
PreOrder(T->rchild); // 遍历右子树
}
}
```
中序遍历:
```c++
void InOrder(BiTree T) {
if(T != NULL) {
InOrder(T->lchild); // 遍历左子树
printf("%c", T->data); // 访问根节点
InOrder(T->rchild); // 遍历右子树
}
}
```
后序遍历:
```c++
void PostOrder(BiTree T) {
if(T != NULL) {
PostOrder(T->lchild); // 遍历左子树
PostOrder(T->rchild); // 遍历右子树
printf("%c", T->data); // 访问根节点
}
}
```
2)统计度为1结点的个数的C++代码:
```c++
int CountDegree1(BiTree T) {
if(T == NULL) {
return 0;
}
int count = 0;
if(T->lchild != NULL && T->rchild == NULL) { // 左子树不为空,右子树为空,度为1
count++;
}
if(T->lchild == NULL && T->rchild != NULL) { // 左子树为空,右子树不为空,度为1
count++;
}
count += CountDegree1(T->lchild); // 统计左子树中度为1的节点个数
count += CountDegree1(T->rchild); // 统计右子树中度为1的节点个数
return count;
}
```
相关推荐
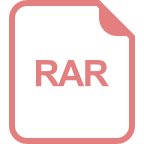
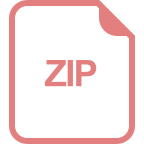
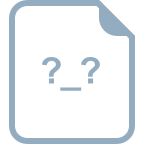















