算法设计题:二叉树采用二叉链表存储结构,请分别用递归和非递归方法编写算法求二叉树的叶子结点的个数。
时间: 2024-10-24 13:17:48 浏览: 8
在二叉树的二叉链表存储结构中,一个节点可以有0个、1个或2个子节点。为了计算叶子节点的数量,我们可以使用递归和非递归两种方法。
**递归方法:**
递归是一种自然的选择,因为二叉树的每个节点要么是叶子节点(没有子节点),要么有一个或两个非叶子节点。我们可以在函数中检查当前节点是否为空,如果为空则返回0;如果非空,判断其是否有左孩子和右孩子,如果没有,则返回1,表示当前节点是一个叶子节点,然后加上左右子树的叶节点数之和。
```python
def count_leaves_recursive(root):
if root is None:
return 0
elif root.left is None and root.right is None:
return 1
else:
left_leaves = count_leaves_recursive(root.left)
right_leaves = count_leaves_recursive(root.right)
return left_leaves + right_leaves
```
**非递归方法(迭代):**
非递归方法通常需要借助栈来模拟递归过程。我们从根节点开始,将所有遇到的叶子节点压入栈中,直到栈为空。当遇到叶子节点时,只需计数即可。
```python
def count_leaves_iterative(root):
leaf_count = 0
stack = [root]
while stack:
node = stack.pop()
if node.left is None and node.right is None:
leaf_count += 1
if node.right:
stack.append(node.right)
if node.left:
stack.append(node.left)
return leaf_count
```
阅读全文
相关推荐
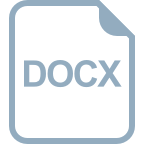
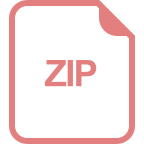















