python实现随机从文件中读取不重复的图片
时间: 2024-05-11 10:20:18 浏览: 6
可以按照以下步骤实现随机从文件中读取不重复的图片:
1. 导入`os`和`random`模块。`os`模块用于获取文件夹中的文件列表,`random`模块用于生成随机数。
```python
import os
import random
```
2. 定义一个函数,用于获取文件夹中的图片文件列表。这里假设图片文件的扩展名为`.jpg`,你可以根据实际情况进行修改。
```python
def get_image_list(folder_path):
image_list = []
for filename in os.listdir(folder_path):
if filename.endswith('.jpg'):
image_list.append(os.path.join(folder_path, filename))
return image_list
```
3. 在主程序中调用`get_image_list`函数获取图片文件列表,并使用`random.sample`函数从列表中随机选择一个元素。`random.sample`函数可以确保所选元素不会重复。
```python
folder_path = 'path/to/your/folder'
image_list = get_image_list(folder_path)
image_path = random.sample(image_list, 1)[0]
```
4. 加载并显示所选图片。这里可以使用Pillow库中的`Image`模块。
```python
from PIL import Image
image = Image.open(image_path)
image.show()
```
完整的代码如下:
```python
import os
import random
from PIL import Image
def get_image_list(folder_path):
image_list = []
for filename in os.listdir(folder_path):
if filename.endswith('.jpg'):
image_list.append(os.path.join(folder_path, filename))
return image_list
folder_path = 'path/to/your/folder'
image_list = get_image_list(folder_path)
image_path = random.sample(image_list, 1)[0]
image = Image.open(image_path)
image.show()
```
相关推荐
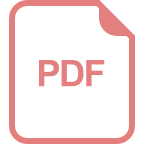
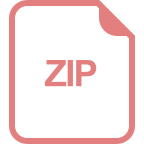
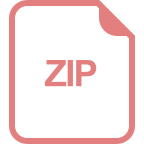














