no = na * (nc + 5) # number of outputs = anchors * (classes + 5)
时间: 2024-04-06 22:34:50 浏览: 112
这段代码的作用是计算输出层的节点数。在目标检测任务中,输出层通常由一个密集连接的层组成,其输出节点数由 anchor box 数量和类别数量确定。在这段代码中,na 是 anchor box 的数量,nc 是目标类别的数量。因此,no 将被计算为 na 乘以 (nc + 5),其中 5 表示每个 anchor box 需要预测的 5 个值:偏移量、置信度和目标类别的概率分布。因此,这段代码将返回输出层所需的节点数。
相关问题
class Detect(nn.Module): stride = None # strides computed during build onnx_dynamic = False # ONNX export parameter def init(self, nc=80, anchors=(), ch=(), inplace=True): # detection layer super().init() self.nc = nc # number of classes self.no = nc + 5 # number of outputs per anchor self.nl = len(anchors) # number of detection layers self.na = len(anchors[0]) // 2 # number of anchors self.grid = [torch.zeros(1)] * self.nl # init grid a = torch.tensor(anchors).float().view(self.nl, -1, 2) self.register_buffer('anchors', a) # shape(nl,na,2) self.register_buffer('anchor_grid', a.clone().view(self.nl, 1, -1, 1, 1, 2)) # shape(nl,1,na,1,1,2) self.m = nn.ModuleList(nn.Conv2d(x, self.no * self.na, 1) for x in ch) # output conv self.inplace = inplace # use in-place ops (e.g. slice assignment) def forward(self, x): z = [] # inference output for i in range(self.nl): x[i] = self.mi # conv bs, _, ny, nx = x[i].shape # x(bs,255,20,20) to x(bs,3,20,20,85) x[i] = x[i].view(bs, self.na, self.no, ny, nx).permute(0, 1, 3, 4, 2).contiguous() if not self.training: # inference if self.grid[i].shape[2:4] != x[i].shape[2:4] or self.onnx_dynamic: self.grid[i] = self._make_grid(nx, ny).to(x[i].device) y = x[i].sigmoid() if self.inplace: y[..., 0:2] = (y[..., 0:2] * 2. - 0.5 + self.grid[i]) * self.stride[i] # xy y[..., 2:4] = (y[..., 2:4] * 2) ** 2 * self.anchor_grid[i] # wh else: # for YOLOv5 on AWS Inferentia https://github.com/ultralytics/yolov5/pull/2953 xy = (y[..., 0:2] * 2. - 0.5 + self.grid[i]) * self.stride[i] # xy wh = (y[..., 2:4] * 2) ** 2 * self.anchor_grid[i].view(1, self.na, 1, 1, 2) # wh y = torch.cat((xy, wh, y[..., 4:]), -1) z.append(y.view(bs, -1, self.no)) return x if self.training else (torch.cat(z, 1), x) @staticmethod def _make_grid(nx=20, ny=20): yv, xv = torch.meshgrid([torch.arange(ny), torch.arange(nx)]) return torch.stack((xv, yv), 2).view((1, 1, ny, nx, 2)).float() 基于YOLOv5详细介绍这个程序
这段程序是YOLOv5中的检测层(Detect)的实现代码。下面对其中的关键部分进行详细介绍:
1. 初始化函数 (init)
在初始化函数中,定义了一些模型的参数,如类别数(nc)、anchor框(anchors)、通道数(ch)等。同时,还初始化了一些变量,如anchor框的数量(na)、输出的维度数(no)等。在该函数中,还注册了一些buffer,如anchors、anchor_grid等,用于存储一些不需要训练的参数。
2. 前向传播函数 (forward)
在前向传播函数中,首先将输入的特征图(x)通过一些卷积层(m)进行处理,得到一些预测结果(y)。然后,将预测结果进行reshape操作,得到(bs,3,ny,nx,85)的形式,其中bs为batch size,3为每个像素点对应的anchor框数量,ny和nx为特征图的大小,85为每个anchor框的预测结果,包括4个坐标值、1个置信度值和80个类别概率值。
接着,根据预测的坐标值和anchor框计算出每个目标的边界框,并根据置信度值和类别概率值进行预测。具体来说,对于每个像素点和每个anchor框,根据预测的中心坐标、宽度和高度,计算出对应的边界框。然后,根据预测的置信度值和类别概率值,对每个边界框进行分类,得到最终的目标检测结果。
在推理过程中,为了适应不同大小的输入图像,检测层还支持动态形状的输入输出。此外,为了提高推理速度,该模型还使用了一些技巧,如in-place操作、buffer注册等。
class Detect(nn.Module): stride = None # strides computed during build onnx_dynamic = False # ONNX export parameter def __init__(self, nc=80, anchors=(), ch=(), inplace=True): # detection layer super().__init__() self.nc = nc # number of classes self.no = nc + 5 # number of outputs per anchor self.nl = len(anchors) # number of detection layers self.na = len(anchors[0]) // 2 # number of anchors self.grid = [torch.zeros(1)] * self.nl # init grid a = torch.tensor(anchors).float().view(self.nl, -1, 2) self.register_buffer('anchors', a) # shape(nl,na,2) self.register_buffer('anchor_grid', a.clone().view(self.nl, 1, -1, 1, 1, 2)) # shape(nl,1,na,1,1,2) self.m = nn.ModuleList(nn.Conv2d(x, self.no * self.na, 1) for x in ch) # output conv self.inplace = inplace # use in-place ops (e.g. slice assignment) def forward(self, x): z = [] # inference output for i in range(self.nl): x[i] = self.m[i](x[i]) # conv bs, _, ny, nx = x[i].shape # x(bs,255,20,20) to x(bs,3,20,20,85) x[i] = x[i].view(bs, self.na, self.no, ny, nx).permute(0, 1, 3, 4, 2).contiguous() if not self.training: # inference if self.grid[i].shape[2:4] != x[i].shape[2:4] or self.onnx_dynamic: self.grid[i] = self._make_grid(nx, ny).to(x[i].device) y = x[i].sigmoid() if self.inplace: y[..., 0:2] = (y[..., 0:2] * 2. - 0.5 + self.grid[i]) * self.stride[i] # xy y[..., 2:4] = (y[..., 2:4] * 2) ** 2 * self.anchor_grid[i] # wh else: # for YOLOv5 on AWS Inferentia https://github.com/ultralytics/yolov5/pull/2953 xy = (y[..., 0:2] * 2. - 0.5 + self.grid[i]) * self.stride[i] # xy wh = (y[..., 2:4] * 2) ** 2 * self.anchor_grid[i].view(1, self.na, 1, 1, 2) # wh y = torch.cat((xy, wh, y[..., 4:]), -1) z.append(y.view(bs, -1, self.no)) return x if self.training else (torch.cat(z, 1), x) @staticmethod def _make_grid(nx=20, ny=20): yv, xv = torch.meshgrid([torch.arange(ny), torch.arange(nx)]) return torch.stack((xv, yv), 2).view((1, 1, ny, nx, 2)).float()
这是一个 PyTorch 中的 Detect 模块的定义。它是 YOLOv5 模型的核心组成部分之一,用于检测图像中的目标,并输出相应的边界框、置信度和类别信息。
具体来说,这个模块包含以下几个主要组成部分:
1. 初始化函数:定义了模块的一些基本参数,如目标的类别数、锚框的数量、输出通道数等。
2. 前向传播函数:接收输入张量 x,经过一系列卷积操作后,输出检测结果。其中,这个函数包含以下几个主要步骤:
a. 对于每个 detection 层,将输入张量 x 经过一个 1x1 的卷积层,得到输出张量;
b. 将输出张量的形状转换为 (batch_size, num_anchors, num_outputs, H, W) 的形式;
c. 对于每个锚框,计算其对应的边界框的位置和置信度等信息;
d. 将不同 detection 层的检测结果拼接起来,得到最终的检测结果。
3. 辅助函数 _make_grid:用于生成网格坐标,用于计算锚框的位置信息。
总的来说,这个 Detect 模块实现了 YOLOv5 检测算法的核心逻辑,是 YOLOv5 模型的重要组成部分之一。
阅读全文
相关推荐
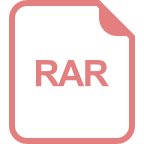
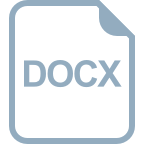
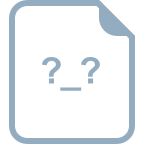





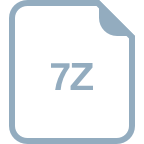
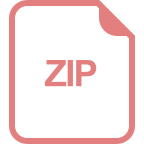