std::string 在C#中如何调用
时间: 2023-11-13 07:05:04 浏览: 269
C# 中没有 std::string 类,它使用的是 System.String 类。System.String 类似乎是在 C++ 中的 std::string 类和 C# 中的 String 类之间进行了合并。您可以使用 System.String 类来表示和操作 C# 中的字符串。
在 C# 中,您可以使用以下方式创建和操作字符串:
```csharp
// 创建一个字符串变量
string myString = "Hello, world!";
// 获取字符串长度
int length = myString.Length;
// 获取子字符串
string subString = myString.Substring(7);
// 拼接两个字符串
string newString = myString + " This is a new string.";
// 查找子字符串的位置
int index = myString.IndexOf("world");
// 替换子字符串
string replacedString = myString.Replace("world", "universe");
```
希望这可以帮助您在 C# 中使用字符串。如果您有任何其他问题,请随时问我。
相关问题
swig 转换std::string&为c#string
SWIG是一个软件开发工具,用于连接C/C++代码和其他高级编程语言。在使用SWIG将C++库封装到C#中时,SWIG需要知道如何将C++的数据类型转换为C#的数据类型。对于std::string类,SWIG提供了一种机制来自动处理std::string与C#的System.String之间的转换。
为了确保SWIG能够正确地将C++中的std::string引用转换为C#中的string类型,需要在SWIG的接口文件(.i文件)中声明std::string与System.String之间的转换。具体来说,需要使用SWIG的typemap功能来定义转换规则。下面是一个简单的例子来说明如何在SWIG接口文件中设置这种转换:
```swig
// 在SWIG接口文件中
%module example
%{
#include <string>
%}
%include <std_string.i> // 引入SWIG预定义的std::string typemaps
%inline %{
std::string foo(const std::string& str) {
return str + " processed";
}
%}
```
在这个例子中,通过 `%include <std_string.i>` 指令,SWIG会自动包含用于std::string类型的typemaps,这些typemaps提供了std::string到C# string之间的自动转换规则。然后,你可以在SWIG封装的代码中直接使用std::string作为参数或者返回类型,而无需担心手动转换问题。
在C#中调用封装后的函数时,SWIG会处理好std::string与System.String之间的转换,使得C#代码可以像使用C#内置类型一样使用这些函数。
c++ 调用c# dll System::String^转为std::string
在C++中调用C# DLL并转换`System::String^`类型的字符串为`std::string`,你需要借助一些第三方库,如PInvoke (Platform Invoke) 或者 C++/CLI(Common Language Infrastructure for CLI)。以下是一个基本的步骤:
1. 包含头文件:首先,你需要包含`clr`库,这通常是在Windows平台上使用的。
```cpp
#include <windows.h>
using namespace std;
using namespace System;
using namespace System::Runtime.InteropServices;
```
2. 定义DllImport:声明你要调用的DLL函数,并指定返回类型和参数类型,包括`System::String^`。
```cpp
[DllImport("YourDLLName.dll", CallingConvention = CallingConvention.Cdecl)]
extern "C" string^ GetCSharpString();
```
这里的`YourDLLName.dll`需要替换为实际的DLL文件名。
3. 转换方法:创建一个函数来处理从C#传回的`System::String^`并将它转换成`std::string`。你可以使用`marshal_as`来完成转换。
```cpp
string ConvertToStdString(System::String^ str)
{
return marshal_as<std::string>(str);
}
```
4. 调用C#方法并转换结果:
```cpp
std::string result = ConvertToStdString(GetCSharpString());
```
注意:这个过程需要在.NET Framework环境中运行,并且不是跨语言的通用解决方案,因为C++和C#有本质的语言差异。
阅读全文
相关推荐
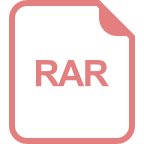






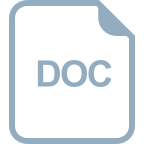
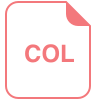






