使用c语言写一个能让bmp位图旋转给定角度的程序,bmp位图命名为data.bmp,旋转后的图像命名为datar.bmp
时间: 2024-04-12 13:32:05 浏览: 25
好的,以下是一个使用C语言编写的程序,可以将给定角度的BMP位图旋转,并将旋转后的图像保存为datar.bmp。
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
typedef struct {
unsigned char blue;
unsigned char green;
unsigned char red;
} Pixel;
typedef struct {
int width;
int height;
Pixel *data;
} Image;
void rotateImage(Image *src, Image *dst, double angle) {
double radian = angle * M_PI / 180.0;
double cosValue = cos(radian);
double sinValue = sin(radian);
int cx = src->width / 2;
int cy = src->height / 2;
for (int y = 0; y < dst->height; y++) {
for (int x = 0; x < dst->width; x++) {
int srcX = (int)(cosValue * (x - cx) + sinValue * (y - cy) + cx);
int srcY = (int)(-sinValue * (x - cx) + cosValue * (y - cy) + cy);
if (srcX >= 0 && srcX < src->width && srcY >= 0 && srcY < src->height) {
int dstIndex = y * dst->width + x;
int srcIndex = srcY * src->width + srcX;
dst->data[dstIndex] = src->data[srcIndex];
}
}
}
}
Image *readBmp(const char *filename) {
FILE *file = fopen(filename, "rb");
if (!file) {
printf("Error: Failed to open file '%s'\n", filename);
return NULL;
}
unsigned char header[54];
if (fread(header, sizeof(unsigned char), 54, file) != 54) {
printf("Error: Invalid BMP file\n");
fclose(file);
return NULL;
}
int width = *(int*)&header[18];
int height = *(int*)&header[22];
int dataSize = *(int*)&header[34];
if (dataSize == 0) {
dataSize = width * height * 3;
}
unsigned char *data = (unsigned char*)malloc(dataSize);
if (!data) {
printf("Error: Failed to allocate memory for image data\n");
fclose(file);
return NULL;
}
fread(data, sizeof(unsigned char), dataSize, file);
fclose(file);
Image *image = (Image*)malloc(sizeof(Image));
image->width = width;
image->height = height;
image->data = (Pixel*)malloc(width * height * sizeof(Pixel));
int dataIndex = 0;
for (int i = 0; i < width * height; i++) {
image->data[i].blue = data[dataIndex++];
image->data[i].green = data[dataIndex++];
image->data[i].red = data[dataIndex++];
}
free(data);
return image;
}
void writeBmp(const char *filename, Image *image) {
unsigned char header[54] = {
'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 54, 0, 0, 0, 40, 0, 0, 0,
0, 0, 0, 0, 0, 0, 1, 0, 24, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
};
*(int*)&header[18] = image->width;
*(int*)&header[22] = image->height;
*(int*)&header[34] = image->width * image->height * sizeof(Pixel);
FILE *file = fopen(filename, "wb");
if (!file) {
printf("Error: Failed to create file '%s'\n", filename);
return;
}
fwrite(header, sizeof(unsigned char), 54, file);
for (int i = 0; i < image->width * image->height; i++) {
fwrite(&image->data[i].blue, sizeof(unsigned char), 1, file);
fwrite(&image->data[i].green, sizeof(unsigned char), 1, file);
fwrite(&image->data[i].red, sizeof(unsigned char), 1, file);
}
fclose(file);
}
int main() {
const char *inputFilename = "data.bmp";
const char *outputFilename = "datar.bmp";
double rotationAngle = 45.0;
Image *inputImage = readBmp(inputFilename);
if (!inputImage) {
return -1;
}
Image *outputImage = (Image*)malloc(sizeof(Image));
outputImage->width = inputImage->width;
outputImage->height = inputImage->height;
outputImage->data = (Pixel*)malloc(inputImage->width * inputImage->height * sizeof(Pixel));
rotateImage(inputImage, outputImage, rotationAngle);
writeBmp(outputFilename, outputImage);
free(inputImage->data);
free(inputImage);
free(outputImage->data);
free(outputImage);
return 0;
}
```
请将上述代码保存到一个名为 `bmp_rotation.c` 的文件中,并确保你已经有一个名为 `data.bmp` 的BMP图像文件放在同一目录下。然后,使用C编译器编译运行该程序,它将生成一个名为 `datar.bmp` 的旋转后的图像文件。
请注意,这个程序只支持24位颜色的BMP图像。如果你的图像是其他格式或是压缩过的,可能会出现问题。此外,该程序只能进行整数度数的旋转,如果需要更精确的旋转,你可能需要使用更高级的算法或库。
相关推荐
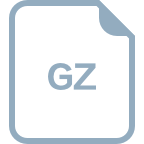
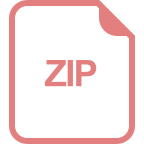





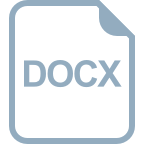
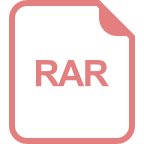
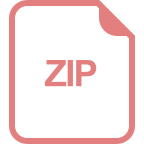
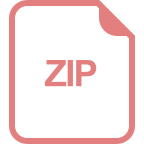
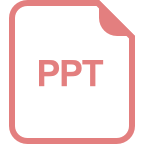
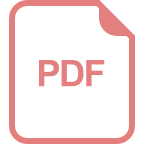