帮我编写一个程序,要求如下,给出完整定义的代码 1、先序遍历二叉树的递归算法 2、中序遍历二叉树的递归算法 3、后序遍历二叉树的递归算法 4、计算二叉树的深度的递归算法 5、统计二叉树的结点个数的递归算法 6、统计二叉树的叶子结点个数的递归算法 7、设计该二叉树第K层的结点个数。 8、求该二叉树中所有结点值最大的元素。 9、打印二叉树的叶子结点数的递归算法 给出完整的程序代码
时间: 2023-11-13 17:25:27 浏览: 71
好的,以下是完整的程序代码:
```c++
#include <iostream>
using namespace std;
// 二叉树节点的定义
struct TreeNode {
int val;
TreeNode *left;
TreeNode *right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
// 先序遍历二叉树的递归算法
void preorderTraversal(TreeNode* root) {
if (root == NULL) return;
cout << root->val << " ";
preorderTraversal(root->left);
preorderTraversal(root->right);
}
// 中序遍历二叉树的递归算法
void inorderTraversal(TreeNode* root) {
if (root == NULL) return;
inorderTraversal(root->left);
cout << root->val << " ";
inorderTraversal(root->right);
}
// 后序遍历二叉树的递归算法
void postorderTraversal(TreeNode* root) {
if (root == NULL) return;
postorderTraversal(root->left);
postorderTraversal(root->right);
cout << root->val << " ";
}
// 计算二叉树的深度的递归算法
int maxDepth(TreeNode* root) {
if (root == NULL) return 0;
int leftDepth = maxDepth(root->left);
int rightDepth = maxDepth(root->right);
return max(leftDepth, rightDepth) + 1;
}
// 统计二叉树的结点个数的递归算法
int countNodes(TreeNode* root) {
if (root == NULL) return 0;
return countNodes(root->left) + countNodes(root->right) + 1;
}
// 统计二叉树的叶子结点个数的递归算法
int countLeaves(TreeNode* root) {
if (root == NULL) return 0;
if (root->left == NULL && root->right == NULL) return 1;
return countLeaves(root->left) + countLeaves(root->right);
}
// 设计该二叉树第K层的结点个数
int countNodesInKthLevel(TreeNode* root, int k) {
if (root == NULL || k < 1) return 0;
if (k == 1) return 1;
return countNodesInKthLevel(root->left, k - 1) + countNodesInKthLevel(root->right, k - 1);
}
// 求该二叉树中所有结点值最大的元素
int findMax(TreeNode* root) {
if (root == NULL) return INT_MIN;
int leftMax = findMax(root->left);
int rightMax = findMax(root->right);
return max(root->val, max(leftMax, rightMax));
}
// 打印二叉树的叶子结点数的递归算法
void printLeaves(TreeNode* root) {
if (root == NULL) return;
if (root->left == NULL && root->right == NULL) cout << root->val << " ";
printLeaves(root->left);
printLeaves(root->right);
}
// 示例代码
int main() {
TreeNode* root = new TreeNode(1);
root->left = new TreeNode(2);
root->right = new TreeNode(3);
root->left->left = new TreeNode(4);
root->left->right = new TreeNode(5);
root->right->left = new TreeNode(6);
root->right->right = new TreeNode(7);
cout << "先序遍历:";
preorderTraversal(root);
cout << endl;
cout << "中序遍历:";
inorderTraversal(root);
cout << endl;
cout << "后序遍历:";
postorderTraversal(root);
cout << endl;
cout << "深度为:" << maxDepth(root) << endl;
cout << "结点个数为:" << countNodes(root) << endl;
cout << "叶子结点个数为:" << countLeaves(root) << endl;
cout << "第3层的结点个数为:" << countNodesInKthLevel(root, 3) << endl;
cout << "节点值最大的元素为:" << findMax(root) << endl;
cout << "叶子结点的值为:";
printLeaves(root);
cout << endl;
return 0;
}
```
以上是完整的程序代码,你可以直接在 C++ 编译器中运行。注意要先定义二叉树节点的结构体 `TreeNode`,然后实现每个函数的具体逻辑。
阅读全文
相关推荐
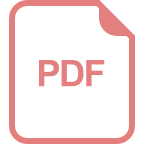
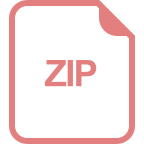
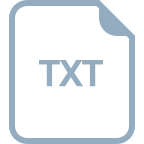
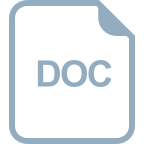
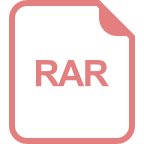
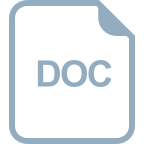
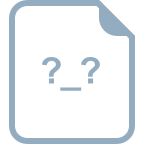
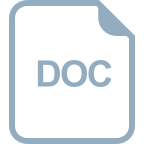
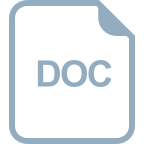
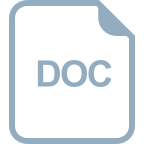
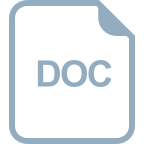

