帮我补充下面的代码:linknode *ptr = head->next; while (ptr != NULL) { if (ptr->flag == 0 && ptr->needMemory <= memory && ptr->needTape <= tape) { //如果作业未调度,并且系统资源足够,则将该作业调入就绪队列 ptr->flag = 1; ptr->jobTime = now_time; memory -= ptr->needMemory; tape -= ptr->needTape; } ptr = ptr->next; }
时间: 2024-02-14 19:17:07 浏览: 74
这是一个遍历链表的代码段,并且在遍历的过程中查找符合条件的作业并将其调入就绪队列。接下来,你可以在这段代码之后添加其他的逻辑来实现作业调度。例如,你可以将符合条件的作业加入到就绪队列中,然后按照一定的调度算法从就绪队列中选择一个作业来执行。下面是一个可能的代码示例:
```c
linknode *ptr = head->next;
while (ptr != NULL) {
if (ptr->flag == 0 && ptr->needMemory <= memory && ptr->needTape <= tape) {
//如果作业未调度,并且系统资源足够,则将该作业调入就绪队列
ptr->flag = 1;
ptr->jobTime = now_time;
memory -= ptr->needMemory;
tape -= ptr->needTape;
//将该作业加入到就绪队列中
add_job_to_ready_queue(ptr);
}
ptr = ptr->next;
}
//从就绪队列中选择一个作业来执行
schedule_job_from_ready_queue();
```
在上面的代码示例中,`add_job_to_ready_queue` 和 `schedule_job_from_ready_queue` 分别表示将作业加入到就绪队列中和从就绪队列中选择一个作业来执行的函数,你需要根据具体的调度算法来实现它们。
相关问题
使用【c++】补全上述代码
由于`createForest`函数的具体实现未提供,这里可以假设一种简单的方式来读取输入字符串并根据字符计数创建森林。我们将使用`std::map`来存储字符及其出现的权重,因为它是C++中查找和更新键值对的理想选择。以下是补全后的`createForest`函数:
```cpp
#include <iostream>
#include <string>
#include <map>
// 创建森林
int createForest(Forest* forest) {
std::string input;
std::cout << "请输入字符串(以 $ 结束):" << std::endl;
getline(std::cin, input); // 获取整个行的输入,包括$
std::map<char, int> frequency; // 存储字符及其出现频率
for (const auto& c : input) {
if (c != '$') { // 避免累加结束符的计数
++frequency[c];
}
}
LinkNode* newNode = forest->next;
for (auto const& [char, count] : frequency) {
newNode = static_cast<LinkNode*>(malloc(sizeof(*newNode)));
newNode->tree = new BiTNode;
newNode->tree->data = char;
newNode->tree->weight = count;
newNode->tree->lchild = newNode->tree->rchild = nullptr;
newNode->next = newNode + 1; // 指向下一个空位置(为了方便后续添加)
newNode++;
forest->next = newNode; // 更新森林的头结点链表
}
return frequency.size(); // 返回森林中树的数量
}
```
接下来,在`main`函数里调用新的`createForest`函数:
```cpp
int main() {
Forest forest = malloc(sizeof(linkNode));
forest->tree = malloc(sizeof(BiTNode));
forest->tree->data = '$';
forest->next = NULL;
int numTrees = createForest(&forest);
char ch1;
scanf("%c", &ch1);
LinkNode* p = forest->next;
while (p != NULL) {
if (p->tree->data == ch1) {
printf("%c:%d\n", p->tree->data, p->tree->weight);
}
p = p->next;
}
return 0;
}
```
现在,这个版本的程序可以读取用户输入的字符串,计算每个字符的出现频率,并根据频率创建森林。注意,`malloc`在C++11之后应该使用智能指针`unique_ptr`或`shared_ptr`代替,但此处为了简洁起见未做转换。
阅读全文
相关推荐
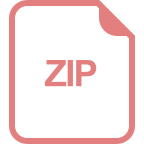
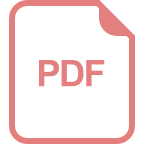
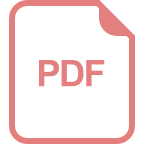
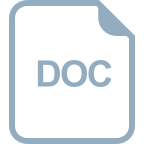
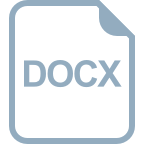
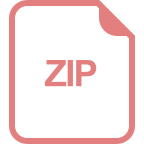
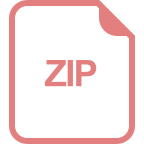
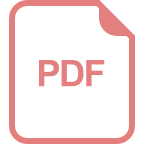