inception10滚动轴承故障诊断数据集代码
时间: 2024-06-09 14:08:22 浏览: 168
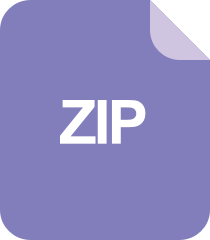
根据轴承的振动序列数据来诊断轴承故障(python代码)
以下是使用TensorFlow和Keras实现的Inception10模型来进行滚动轴承故障诊断的代码。
首先,我们需要加载数据集。可以在此处下载数据集:https://data.mendeley.com/datasets/bxk2sdx3wy/1
```python
import numpy as np
import os
# 加载数据集
def load_dataset():
dataset_path = "data/"
train_X = np.load(os.path.join(dataset_path, "train_X.npy"))
train_y = np.load(os.path.join(dataset_path, "train_y.npy"))
test_X = np.load(os.path.join(dataset_path, "test_X.npy"))
test_y = np.load(os.path.join(dataset_path, "test_y.npy"))
return train_X, train_y, test_X, test_y
```
接下来,我们需要构建Inception10模型。在这里,我们使用了Inception模块,这是Google在2014年提出的一种卷积神经网络结构。
```python
from tensorflow.keras import layers, models
# 构建Inception模块
def inception_module(inputs, filters):
path1 = layers.Conv2D(filters=filters[0], kernel_size=1, activation="relu")(inputs)
path2 = layers.Conv2D(filters=filters[1], kernel_size=1, activation="relu")(inputs)
path2 = layers.Conv2D(filters=filters[2], kernel_size=3, padding="same", activation="relu")(path2)
path3 = layers.Conv2D(filters=filters[3], kernel_size=1, activation="relu")(inputs)
path3 = layers.Conv2D(filters=filters[4], kernel_size=5, padding="same", activation="relu")(path3)
path4 = layers.MaxPooling2D(pool_size=3, strides=1, padding="same")(inputs)
path4 = layers.Conv2D(filters=filters[5], kernel_size=1, activation="relu")(path4)
return layers.concatenate([path1, path2, path3, path4])
# 构建Inception10模型
def build_inception10(input_shape, num_classes):
inputs = layers.Input(shape=input_shape)
x = layers.Conv2D(filters=64, kernel_size=7, strides=2, padding="same", activation="relu")(inputs)
x = layers.MaxPooling2D(pool_size=3, strides=2, padding="same")(x)
x = layers.Conv2D(filters=64, kernel_size=1, activation="relu")(x)
x = layers.Conv2D(filters=192, kernel_size=3, padding="same", activation="relu")(x)
x = layers.MaxPooling2D(pool_size=3, strides=2, padding="same")(x)
x = inception_module(x, filters=[64, 96, 128, 16, 32, 32])
x = inception_module(x, filters=[128, 128, 192, 32, 96, 64])
x = layers.MaxPooling2D(pool_size=3, strides=2, padding="same")(x)
x = inception_module(x, filters=[192, 96, 208, 16, 48, 64])
x = inception_module(x, filters=[160, 112, 224, 24, 64, 64])
x = inception_module(x, filters=[128, 128, 256, 24, 64, 64])
x = inception_module(x, filters=[112, 144, 288, 32, 64, 64])
x = inception_module(x, filters=[256, 160, 320, 32, 128, 128])
x = layers.MaxPooling2D(pool_size=3, strides=2, padding="same")(x)
x = inception_module(x, filters=[256, 160, 320, 32, 128, 128])
x = inception_module(x, filters=[384, 192, 384, 48, 128, 128])
x = layers.GlobalAveragePooling2D()(x)
x = layers.Dropout(0.4)(x)
outputs = layers.Dense(num_classes, activation="softmax")(x)
return models.Model(inputs, outputs)
```
然后,我们可以定义一些超参数并训练模型。
```python
from tensorflow.keras import optimizers, losses, metrics
from tensorflow.keras.callbacks import ModelCheckpoint, EarlyStopping
# 定义超参数
input_shape = (64, 64, 1)
num_classes = 4
batch_size = 128
epochs = 50
# 加载数据集
train_X, train_y, test_X, test_y = load_dataset()
# 构建模型
model = build_inception10(input_shape, num_classes)
# 编译模型
model.compile(optimizer=optimizers.Adam(), loss=losses.sparse_categorical_crossentropy, metrics=[metrics.sparse_categorical_accuracy])
# 定义回调函数
callbacks = [
ModelCheckpoint("inception10.h5", save_best_only=True),
EarlyStopping(patience=5, restore_best_weights=True)
]
# 训练模型
history = model.fit(train_X, train_y, batch_size=batch_size, epochs=epochs, validation_data=(test_X, test_y), callbacks=callbacks)
# 评估模型
loss, accuracy = model.evaluate(test_X, test_y)
print("Test loss: {:.4f}, accuracy: {:.4f}".format(loss, accuracy))
```
最后,我们可以使用模型进行预测。
```python
# 使用模型进行预测
predictions = model.predict(test_X)
# 输出预测结果
print(predictions)
```
阅读全文
相关推荐
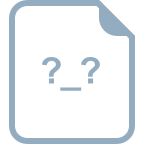
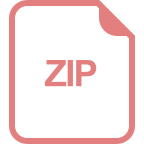

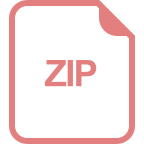

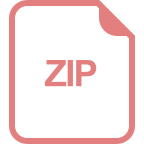
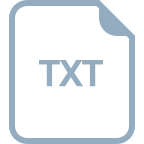
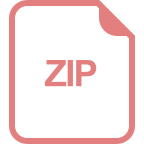
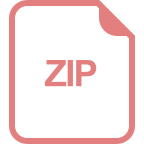
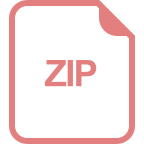
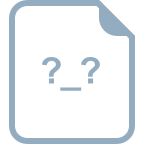
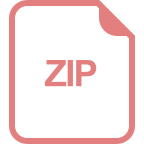
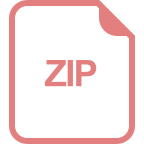

