three tween 做飞线效果
时间: 2024-02-11 07:57:24 浏览: 28
Three.js 中的 Tween 功能可以用于创建动画效果。飞线效果可以通过以下步骤实现:
1. 创建一个空的 THREE.Group 对象,并将其用作飞线的起点和终点。
```
const group = new THREE.Group();
scene.add(group);
```
2. 创建一个 THREE.Curve 对象,用于定义一条曲线。这条曲线将作为飞线的路径。
```
const curve = new THREE.CatmullRomCurve3([
new THREE.Vector3(-10, 0, 0),
new THREE.Vector3(0, 10, 0),
new THREE.Vector3(10, 0, 0)
]);
```
3. 创建一个 THREE.Geometry 对象,并将其用作飞线的形状。在这个例子中,我们使用一个 CylinderGeometry 对象。
```
const geometry = new THREE.CylinderGeometry(0.5, 0.5, 10);
```
4. 创建一个 THREE.Mesh 对象,并将其用作飞线的外观。将其位置设置为曲线的起点。
```
const material = new THREE.MeshBasicMaterial({ color: 0xffffff });
const mesh = new THREE.Mesh(geometry, material);
mesh.position.copy(curve.getPoint(0));
group.add(mesh);
```
5. 创建一个 Tween 动画,将飞线沿着曲线移动。在 Tween 的 onUpdate 回调函数中,更新飞线的位置和旋转。
```
const tween = new TWEEN.Tween({ t: 0 })
.to({ t: 1 }, 3000)
.onUpdate(() => {
const position = curve.getPoint(tween._object.t);
mesh.position.copy(position);
mesh.lookAt(curve.getPoint(tween._object.t + 0.01));
})
.start();
```
完整的代码示例如下:
```
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(60, window.innerWidth / window.innerHeight, 0.1, 100);
camera.position.set(0, 0, 20);
const renderer = new THREE.WebGLRenderer({ antialias: true });
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
const group = new THREE.Group();
scene.add(group);
const curve = new THREE.CatmullRomCurve3([
new THREE.Vector3(-10, 0, 0),
new THREE.Vector3(0, 10, 0),
new THREE.Vector3(10, 0, 0)
]);
const geometry = new THREE.CylinderGeometry(0.5, 0.5, 10);
const material = new THREE.MeshBasicMaterial({ color: 0xffffff });
const mesh = new THREE.Mesh(geometry, material);
mesh.position.copy(curve.getPoint(0));
group.add(mesh);
const tween = new TWEEN.Tween({ t: 0 })
.to({ t: 1 }, 3000)
.onUpdate(() => {
const position = curve.getPoint(tween._object.t);
mesh.position.copy(position);
mesh.lookAt(curve.getPoint(tween._object.t + 0.01));
})
.start();
function animate() {
requestAnimationFrame(animate);
TWEEN.update();
renderer.render(scene, camera);
}
animate();
```
相关推荐
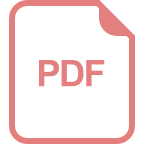
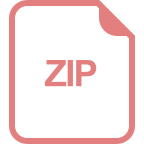














