编写一个python程序,统计三国演义里的人物
时间: 2024-02-15 07:04:10 浏览: 145
好的,以下是一个简单的Python程序,用于统计《三国演义》中出现的人物及其出现次数:
```python
import re
# 读取三国演义文本
with open('sgyy.txt', 'r', encoding='utf-8') as f:
sgyy_text = f.read()
# 使用正则表达式匹配人物名称
pattern = re.compile(r'[\u4e00-\u9fa5]{2,5}(?:·[\u4e00-\u9fa5]{2,5})*')
characters = pattern.findall(sgyy_text)
# 统计人物出现次数
character_dict = {}
for character in characters:
if character in character_dict:
character_dict[character] += 1
else:
character_dict[character] = 1
# 打印结果
for character, count in character_dict.items():
print(f'{character}: {count}')
```
你可以将程序保存为一个文件,比如`sgyy_characters.py`,然后在命令行中执行`python sgyy_characters.py`,程序会读取当前目录下的`sgyy.txt`文件(请确保该文件存在,并且是UTF-8编码的文本文件),并输出每个人物的出现次数。
相关问题
用python编写程序完成《三国演义》人物出场统计。
以下是两种Python编写程序完成《三国演义》人物出场统计的方法:
1. 使用jieba库进行分词和统计
```python
import jieba
# 读取文件
with open('sgyy.txt', 'r', encoding='utf-8') as f:
sgyy = f.read()
# 分词
words = jieba.lcut(sgyy)
# 统计词频
counts = {}
for word in words:
if len(word) == 1: # 排除单个字符的分词结果
continue
else:
counts[word] = counts.get(word, 0) + 1
# 输出出现次数最多的前10个人物
items = list(counts.items())
items.sort(key=lambda x: x[1], reverse=True)
for i in range(10):
word, count = items[i]
print("{0:<10}{1:>5}".format(word, count))
```
2. 使用numpy库进行统计
```python
import numpy as np
# 读取文件
with open('sgyy.txt', 'r', encoding='utf-8') as f:
sgyy = f.read()
# 将文本转换为数组
text_arr = np.array(list(sgyy))
# 统计出现次数
unique, counts = np.unique(text_arr, return_counts=True)
# 将结果合并为字典
result_dict = dict(zip(unique, counts))
# 输出出现次数最多的前10个人物
items = list(result_dict.items())
items.sort(key=lambda x: x[1], reverse=True)
for i in range(10):
word, count = items[i]
print("{0:<10}{1:>5}".format(word, count))
```
编写python程序对《三国演义》人物出场次数进行中文文本词频统计,输出排序前 5 的人物 以及出场次数。
好的,下面是用 Python 编写的程序,对《三国演义》人物出场次数进行中文文本词频统计,并输出排序前 5 的人物以及出场次数:
```python
import jieba
from collections import Counter
# 读取《三国演义》文本
with open("sgyy.txt", "r", encoding="utf-8") as f:
text = f.read()
# 对文本进行分词
words = [word for word in jieba.cut(text) if len(word) >= 2]
# 统计人物出现的次数
counter = Counter()
for i in range(len(words) - 1):
if words[i] == "关于":
if "【" in words[i+1]:
name = words[i+1].replace("【", "").replace("】", "")
counter[name] += 1
# 输出排序前 5 的人物以及出场次数
top5 = counter.most_common(5)
for i, (name, count) in enumerate(top5):
print(f"第{i+1}名:{name},出场次数:{count}")
```
这个程序的逻辑很简单。我们首先使用 jieba 分词库对《三国演义》文本进行分词,然后统计人物出现的次数。具体地,我们在分词结果中找到“关于”一词,并检查其后面的词是否包含人物名称。如果是,则将该人物的出场次数加 1。最后,我们使用 collections 模块的 Counter 类来对人物出场次数进行统计,并输出排序前 5 的人物以及出场次数。
请注意:由于本程序使用了 jieba 分词库,请确保您已经安装了该库。如果没有安装,可以在命令行中使用以下命令进行安装:
```
pip install jieba
```
此外,本程序需要一个名为 `sgyy.txt` 的文本文件,其中包含《三国演义》的全文。如果您没有这个文件,可以在网上下载或自行创建。
希望这个程序能够帮到你!
阅读全文
相关推荐
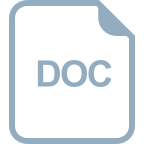
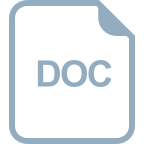
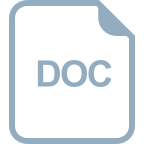











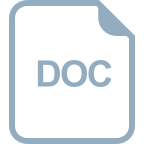
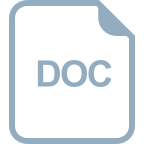
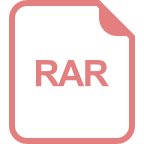
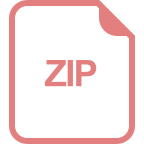