python输入一个正整数n,将文件中的文本读取为字符串,利用jieba库的精确模式将文本切分为字符串,将无实质意义的单字符元素去掉,统计长度大于1的每个字符串出现的频率,存储为字典类型,输出词频最高的元素为二元组的列表前n项。
时间: 2024-02-12 22:07:08 浏览: 27
好的,这是一个比较复杂的问题,需要分步骤来解决。首先,你需要安装jieba库,可以使用以下命令进行安装:
```
pip install jieba
```
接下来,你可以使用以下代码读取文件并进行分词:
```python
import jieba
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as f:
text = f.read()
return text
def cut_words(text):
words = jieba.cut(text, cut_all=False)
return [word for word in words if len(word) > 1]
text = read_file('example.txt')
words = cut_words(text)
```
这段代码中,`read_file()`函数可以读取文件,`cut_words()`函数使用jieba库进行分词,并过滤掉长度小于等于1的单字符元素。
接下来,你需要统计每个字符串出现的频率,并存储为字典类型。可以使用以下代码完成这个任务:
```python
def count_words(words):
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
return word_count
word_count = count_words(words)
```
这段代码中,`count_words()`函数使用字典类型`word_count`来存储每个字符串出现的频率。如果一个字符串已经在字典中出现过,就将其频率加1;否则,将该字符串添加到字典中,并将其频率设为1。
最后,你需要输出词频最高的元素为二元组的列表前n项。可以使用以下代码完成这个任务:
```python
def top_n_words(word_count, n):
sorted_words = sorted(word_count.items(), key=lambda x:x[1], reverse=True)
return sorted_words[:n]
top_n = top_n_words(word_count, n)
print(top_n)
```
这段代码中,`top_n_words()`函数使用`sorted()`函数将字典中的元素按照频率从高到低排序,并返回前n项。最后,你可以使用`print()`函数输出结果。
完整代码如下:
```python
import jieba
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as f:
text = f.read()
return text
def cut_words(text):
words = jieba.cut(text, cut_all=False)
return [word for word in words if len(word) > 1]
def count_words(words):
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
return word_count
def top_n_words(word_count, n):
sorted_words = sorted(word_count.items(), key=lambda x:x[1], reverse=True)
return sorted_words[:n]
text = read_file('example.txt')
words = cut_words(text)
word_count = count_words(words)
top_n = top_n_words(word_count, n)
print(top_n)
```
相关推荐
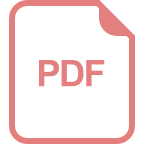
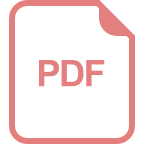
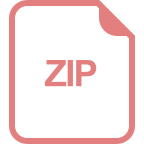














